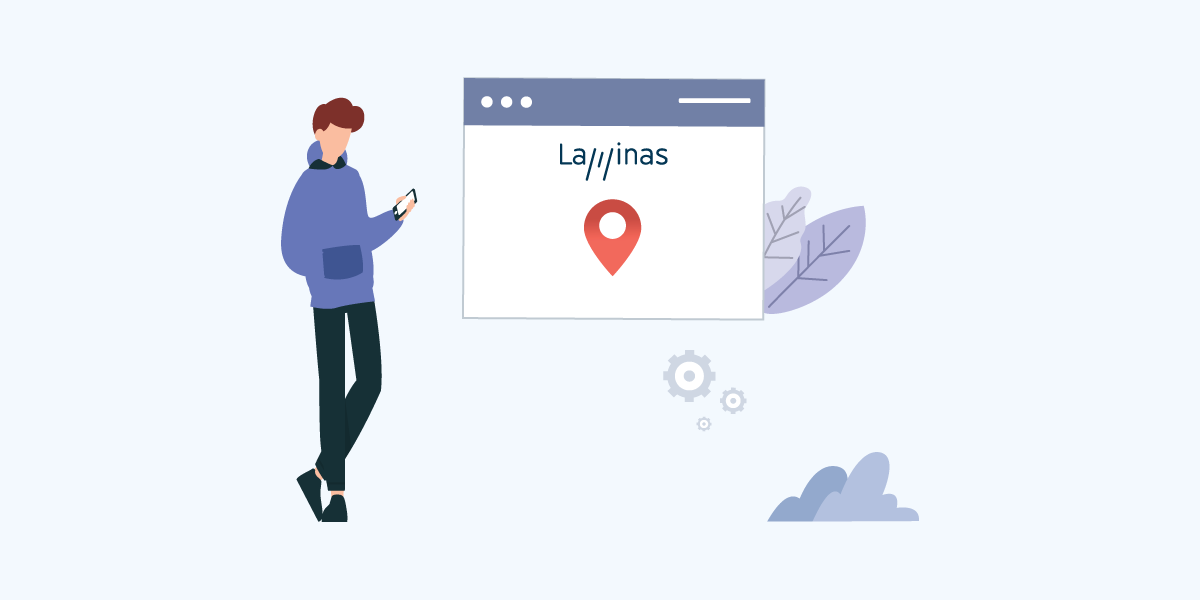
Laminas is a powerful open-source PHP framework for creating web applications. It follows the MVC pattern, promotes code reusability, and integrates with other technical patterns. It provides functionalities like database connectivity, authentication, caching, and form validation while supporting modern PHP practices.
Laminas packages are reusable code modules that can be installed using Composer, a popular PHP package manager. These packages can be found on the Packagist repository and enable easy customization and expansion of functionality in applications.
IP2Location and IP2Location.io PHP SDK
The IP2Location PHP SDK is a software library specifically designed for PHP programming. Its purpose is to assist developers in retrieving geolocation information based on IP addresses.
The package utilizes the IP2Location BIN database, which contains extensive data mapping IP addresses to various geographic details such as country, region, district, city, latitude, longitude, ZIP code, time zone, ISP, domain name, connection speed, IDD code, area code, weather station code, weather station name, MNC, MCC, mobile brand, elevation, usage type, address type, IAB category and ASN. The package supports both IPv4 and IPv6 address lookups, making it suitable for a wide range of PHP projects.
On the other hand, the IP2Location.io PHP SDK is designed for integrating the IP2Location.io geolocation service into PHP applications. It simplifies the retrieval of geolocation information based on IP addresses through a RESTful API.
With the PHP SDK, developers can easily incorporate IP geolocation functionality by querying the API. They can retrieve information such as country, region, district, city, latitude, longitude, ZIP code, time zone, ASN, ISP, domain, net speed, IDD code, area code, weather station data, MNC, MCC, mobile brand, elevation, usage type, address type, advertisement category and proxy data. The SDK eliminates the need for manual HTTP requests and supports both IPv4 and IPv6 address lookups.
Getting Started
In this tutorial, you will learn how to utilize the IP2Location and IP2Location.io PHP SDK within the Laminas framework to obtain geolocation data for display purposes.
To begin with, we assumed that the Laminas framework had been correctly configured prior to following this tutorial. The IP2Location PHP SDK requires the BIN database, however you can obtain a free database from https://lite.ip2location.com/. The functionality of the IP2Location.io PHP SDK relies on an API key, which can be obtained by signing up for a free account at https://www.ip2location.io/pricing. Please be aware that continent, country, region, and city translations are exclusively provided only in the Plus and Security Plans.
Display Geolocation Data Using IP2Location PHP SDK
- Execute the below command to acquire the package and integrate it into the Laminas platform.
composer require ip2location/ip2location-php
- Open the module/Application/src/Controller/IndexController.php in any text editor.
- Add the following codes into the controller file to use IP2Location PHP SDK:
<?php declare(strict_types=1); namespace Application\Controller; use Laminas\Mvc\Controller\AbstractActionController; use Laminas\View\Model\ViewModel; class IndexController extends AbstractActionController { public function indexAction() { // Replace with the IP address you want to geolocate $ipAddress = '8.8.8.8'; // Specify the path to the BIN database file $database = new \IP2Location\Database('path/to/your/IP2LOCATION_BIN_DATABASE.BIN', \IP2Location\Database::FILE_IO); // Retrieve geolocation data $record = $database->lookup($ipAddress, \IP2Location\Database::ALL); // This view model will be returned as the response for the request return new ViewModel([ 'record' => $record, ]); } } ?>
- Open the module/Application/view/application/index/index.phtml in any text editor.
- Add the following codes into the phtml file to display geolocation data:
<?php echo '<pre>'; echo 'IP Number : ' . $record['ipNumber'] . "\n"; echo 'IP Version : ' . $record['ipVersion'] . "\n"; echo 'IP Address : ' . $record['ipAddress'] . "\n"; echo 'Country Code : ' . $record['countryCode'] . "\n"; echo 'Country Name : ' . $record['countryName'] . "\n"; echo 'Region Name : ' . $record['regionName'] . "\n"; echo 'City Name : ' . $record['cityName'] . "\n"; echo 'Latitude : ' . $record['latitude'] . "\n"; echo 'Longitude : ' . $record['longitude'] . "\n"; echo 'Area Code : ' . $record['areaCode'] . "\n"; echo 'IDD Code : ' . $record['iddCode'] . "\n"; echo 'Weather Station Code : ' . $record['weatherStationCode'] . "\n"; echo 'Weather Station Name : ' . $record['weatherStationName'] . "\n"; echo 'MCC : ' . $record['mcc'] . "\n"; echo 'MNC : ' . $record['mnc'] . "\n"; echo 'Mobile Carrier : ' . $record['mobileCarrierName'] . "\n"; echo 'Usage Type : ' . $record['usageType'] . "\n"; echo 'Elevation : ' . $record['elevation'] . "\n"; echo 'Net Speed : ' . $record['netSpeed'] . "\n"; echo 'Time Zone : ' . $record['timeZone'] . "\n"; echo 'ZIP Code : ' . $record['zipCode'] . "\n"; echo 'Domain Name : ' . $record['domainName'] . "\n"; echo 'ISP Name : ' . $record['isp'] . "\n"; echo 'Address Type : ' . ($record['addressType'] ?? 'FIELD NOT SUPPORTED') . "\n"; echo 'Category : ' . ($record['category'] ?? 'FIELD NOT SUPPORTED') . "\n"; echo 'District : ' . ($record['district'] ?? 'FIELD NOT SUPPORTED') . "\n"; echo 'ASN : ' . ($record['asn'] ?? 'FIELD NOT SUPPORTED') . "\n"; echo 'AS : ' . ($record['as'] ?? 'FIELD NOT SUPPORTED') . "\n"; echo '</pre>'; ?>
- Start a web server by running the following command from your project directory in CLI:
php -S localhost:8080 -t public
- Now you can access the application by visiting “http://localhost:8080” in your browser. The page will display the IP geolocation details retrieved using the IP2Location BIN database.
- Done.
Display Geolocation Data Using IP2Location.io PHP SDK
- Execute the below command to acquire the package and integrate it into the Laminas platform.
composer require ip2location/ip2location-io-php
- Open the module/Application/src/Controller/IndexController.php in any text editor.
- Add the following codes into the controller file to use IP2Location.io PHP SDK:
<?php declare(strict_types=1); namespace Application\Controller; use Laminas\Mvc\Controller\AbstractActionController; use Laminas\View\Model\ViewModel; class IndexController extends AbstractActionController { public function indexAction() { // Replace with the IP address you want to geolocate $ipAddress = '8.8.8.8'; // Replace with your actual API key $apiKey = ‘YOUR_API_KEY’; // This class represents the configuration for the IP2Location.io API. $ip2location = new \IP2LocationIO\Configuration($apiKey); // This class is used to perform the geolocation lookup. $ip2locationio = new \IP2LocationIO\IPGeolocation($ip2location); // Sends a request to the IP2Location.io API to retrieve the geolocation information $geolocation = $ip2locationio->lookup($ipAddress); // This view model will be returned as the response for the request return new ViewModel([ 'geolocation' => $geolocation ]); } } ?>
- Open the module/Application/view/application/index/index.phtml in any text editor.
- Add the following codes into the “phtml” file to display geolocation data:
<?php try{ echo 'IP Address : ' . $geolocation->ip . "<br>"; echo 'Country Code : ' . $geolocation->country_code . "<br>"; echo 'Country Name : ' . $geolocation->country_name . "<br>"; echo 'Region Name : ' . $geolocation->region_name . "<br>"; echo 'City Name : ' . $geolocation->city_name . "<br>"; echo 'City Latitude : ' . $geolocation->latitude . "<br>"; echo 'City Longitude : ' . $geolocation->longitude . "<br>"; echo 'ZIP Code : ' . $geolocation->zip_code . "<br>"; echo 'Time Zone : ' . $geolocation->time_zone . "<br>"; echo 'ASN : ' . $geolocation->asn . "<br>"; echo 'AS : ' . $geolocation->as . "<br>"; echo 'Is Proxy : '; echo ($geolocation->is_proxy) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'ISP Name : ' . ($geolocation->isp ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Domain Name : ' . ($geolocation->domain ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Net Speed : ' . ($geolocation->net_speend ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'IDD Code : ' . ($geolocation->idd_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Area Code : ' . ($geolocation->area_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Code : ' . ($geolocation->weather_station_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Name : ' . ($geolocation->weather_station_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MCC : ' . ($geolocation->mcc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MNC : ' . ($geolocation->mnc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Mobile Carrier : ' . ($geolocation->mobile_brand ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Elevation : ' . ($geolocation->elevation ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Usage Type : ' . ($geolocation->usage_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Address Type : ' . ($geolocation->address_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Code : ' . ($geolocation->ads_category ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Name : ' . ($geolocation->ads_category_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'District Name : ' . ($geolocation->district ?? 'FIELD NOT SUPPORTED') . "<br>"; if (isset($geolocation->continent)) { echo 'Continent Name : ' . $geolocation->continent->name . "<br>"; echo 'Continent Code : ' . $geolocation->continent->code . "<br>"; echo 'Continent Hemisphere : '; print_r($geolocation->continent->hemisphere); echo "<br>"; echo 'Continent Translation : ' . $geolocation->continent->translation->value . ' (' . $geolocation->continent->translation->lang . ')' . "<br>"; } if (isset($geolocation->country)) { echo 'Country Name : ' . $geolocation->country->name . "<br>"; echo 'Country Alpha 3 Code : ' . $geolocation->country->alpha3_code . "<br>"; echo 'Country Numeric Code : ' . $geolocation->country->numeric_code . "<br>"; echo 'Country Demonym : ' . $geolocation->country->demonym . "<br>"; echo 'Country Flag : ' . $geolocation->country->flag . "<br>"; echo 'Country Capital : ' . $geolocation->country->capital . "<br>"; echo 'Country Total Area : ' . $geolocation->country->total_area . "<br>"; echo 'Country Population : ' . $geolocation->country->population . "<br>"; echo 'Country Currency Code : ' . $geolocation->country->currency->code . "<br>"; echo 'Country Currency Name : ' . $geolocation->country->currency->name . ' (' . $geolocation->country->currency->symbol . ')' . "<br>"; echo 'Country Language : ' . $geolocation->country->language->name . ' (' . $geolocation->country->language->code . ')' . "<br>"; echo 'Country TLD : ' . $geolocation->country->tld . "<br>"; echo 'Country Translation : ' . $geolocation->country->translation->value . ' (' . $geolocation->country->translation->lang . ')' . "<br>"; } if (isset($geolocation->region)) { echo 'Region Name : ' . $geolocation->region->name . "<br>"; echo 'Region Code : ' . $geolocation->region->code . "<br>"; echo 'Region Translation : ' . $geolocation->region->translation->value . ' (' . $geolocation->region->translation->lang . ')' . "<br>"; } if (isset($geolocation->city)) { echo 'City Name : ' . $geolocation->city->name . "<br>"; echo 'City Translation : ' . $geolocation->city->translation->value . ' (' . $geolocation->city->translation->lang . ')' . "<br>"; } if (isset($geolocation->time_zone_info)) { echo 'Olson Time Zone : ' . $geolocation->time_zone_info->olson . "<br>"; echo 'Current Time : ' . $geolocation->time_zone_info->current_time . "<br>"; echo 'GMT Offset : ' . $geolocation->time_zone_info->gmt_offset . "<br>"; echo 'Is DST : '; echo ($geolocation->time_zone_info->is_dst) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'Sunrise Time : ' . $geolocation->time_zone_info->sunrise . "<br>"; echo 'Sunset Time : ' . $geolocation->time_zone_info->sunset . "<br>"; } if (isset($geolocation->geotargeting)) { echo 'Metro Code : ' . $geolocation->geotargeting->metro . "<br>"; } if (isset($geolocation->proxy)) { echo 'Proxy Last Seen : ' . $geolocation->proxy->last_seen . "<br>"; echo 'Proxy Type : ' . $geolocation->proxy->proxy_type . "<br>"; echo 'Proxy Threat : ' . $geolocation->proxy->threat . "<br>"; echo 'Proxy Provider : ' . $geolocation->proxy->provider . "<br>"; } }catch(Exception $e) { var_dump($e->getCode() . ": " . $e->getMessage()); } ?>
- Start a web server by running the following command from your project directory in CLI:
php -S localhost:8080 -t public/
- Now you can access the application by visiting “http://localhost:8080” in your browser. The page will display the IP geolocation details retrieved using the IP2Location.io API.
- Done.
By the end of this tutorial, you will have the ability to perform an IP geolocation lookup within the Laminas framework using the IP2Location and IP2Location.io PHP SDK. This will allow you to retrieve geolocation data that can be utilized for identifying the user’s geographical details and utilized in data analytics and page redirection.
Learn more on how to use IP2Location.io PHP SDK in various frameworks.