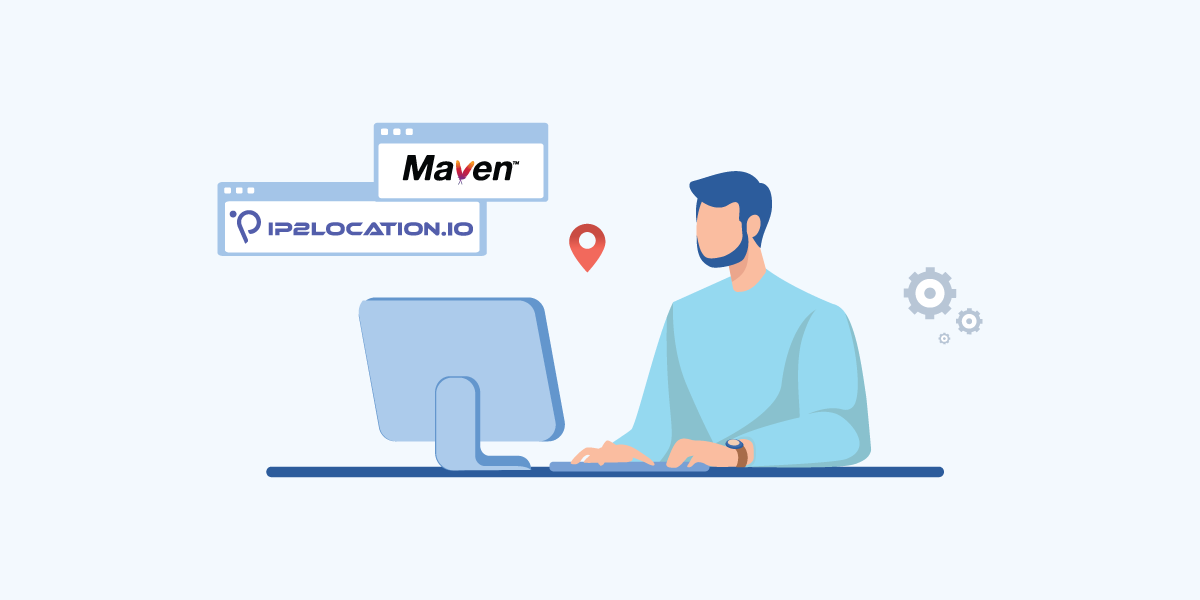
The IP2Location.io Java SDK is a wrapper for 2 APIs; the first being the IP2Location.io Geolocation API and the 2nd being the IP2WHOIS Domain Lookup API. In the article, we’ll cover how to create a new Maven project. Then we’ll add the IP2Location.io Java SDK as a Maven dependency. Finally, we’ll show how run the resulting app to retrieve the data from both APIs. Our demo platform is a Debian 11 machine so all of the steps below will be for that.
NOTE: You will need an IP2Location.io API key so please sign up for one at https://www.ip2location.io/pricing before you proceed further.
Install Java Runtime Environment (JRE) and Java Development Kit (JDK)
Before installing anything, let’s just run the below command to get the latest list of packages available.
sudo apt update
Next, install the JRE with the command below.
sudo apt install default-jre
After that, install the JDK with the command below.
sudo apt install default-jdk
If you need to check the version for the JRE and JDK, you can run the 2 commands below.
java -version

javac -version

Install Maven
Before running the commands below, check at https://maven.apache.org/download.cgi to see what is the latest version of Maven and modify the commands below to use the latest version.
Let’s download Maven using wget.
wget https://dlcdn.apache.org/maven/maven-3/3.9.2/binaries/apache-maven-3.9.2-bin.tar.gz
Extract the folder inside the downloaded file into the /opt folder.
sudo tar xzvf apache-maven-3.9.2-bin.tar.gz -C /opt
You should see the folder inside the /opt folder.

Next, we’ll create a symbolic link to that folder.
sudo ln -s /opt/apache-maven-3.9.2 /opt/maven
Configuring the environment variables required
There are a few environment variables that we need to configure before we can start using Maven.
First of all, we need to determine the path for the java command.
Run the below command to determine the path.
sudo update-alternatives --config java

In our case, the path is /usr/lib/jvm/java-11-openjdk-amd64/bin/java but you may see multiple if your system has multiple versions installed. You can select the one that you wish to use.
Now, run the command below to edit a file called maven.sh where we’ll specify our variables.
sudo nano /etc/profile.d/maven.sh
Paste the below into that file.
export JAVA_HOME=/usr/lib/jvm/java-11-openjdk-amd64 export M2_HOME=/opt/maven export PATH=${M2_HOME}/bin:${PATH}
NOTE: The JAVA_HOME path should NOT include the bin folder part.

After saving the changes in maven.sh, we need to load the variables into the current session.
source /etc/profile.d/maven.sh
Check if Maven has been installed and configured properly.
mvn -version

That’s it for the installation side. Now, we can move on to the coding side.
Creating a new Maven project from a template
Maven has a handy feature to generate a basic Maven project from a template called maven-archetype-quickstart so we don’t have to create all the folders and basic files manually.
First of all, create a folder called Maven_app in your home folder and navigate into it.
mkdir Maven_app cd Maven_app
Next, run the below to generate the basic project.
mvn archetype:generate -DgroupId=com.example.ip2locationio -DartifactId=IP2LocationIOExample -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
In our case, the group id is com.example.ip2locationio and our project is called IP2LocationIOExample.
The created folder structure looks like the below.
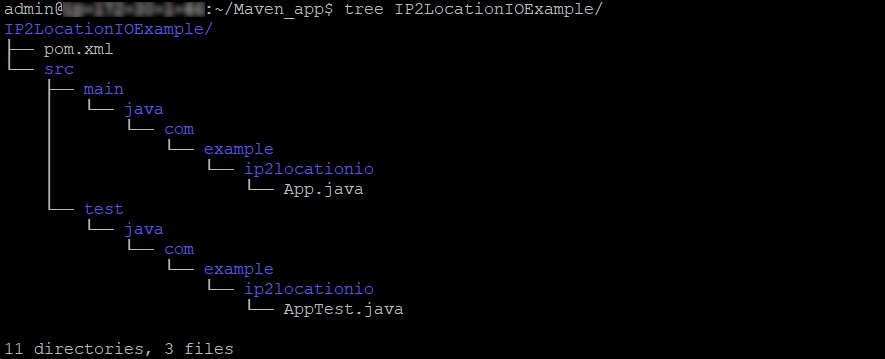
For the purpose of this article, we won’t be doing any test cases, hence we’ll ignore AppTest.java and just modify the pom.xml and App.java files.
Adding the IP2Location.io Java SDK into the Maven dependency
In Maven, all dependencies must be added into the pom.xml file. So, let’s do that now for the IP2Location.io Java SDK. Before that, check and see what is the latest version of the IP2Location.io Java SDK at https://central.sonatype.com/artifact/com.ip2location/ip2location-io-java/ and use the version in the pom.xml below.
Navigate into the IP2LocationIOExample folder and edit the pom.xml file.
cd IP2LocationIOExample nano pom.xml
Add the below into the <dependencies> section and change the version to the latest one.
<dependency> <groupId>com.ip2location</groupId> <artifactId>ip2location-io-java</artifactId> <version>1.0.0</version> </dependency>
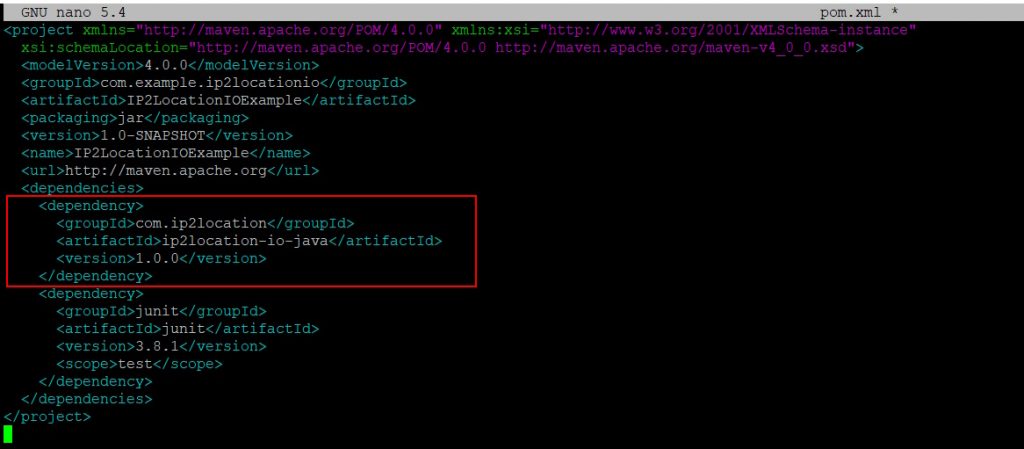
Save your changes.
Modify the App.java file to import and use the IP2Location.io Java SDK
Let’s edit the actual code now.
nano src/main/java/com/example/ip2locationio/App.java
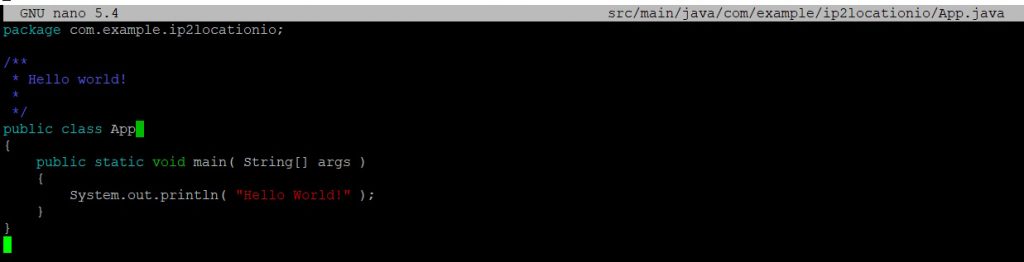
Above is the original code generated by Maven. We’ll modify it to become the below.
Please remember to modify the code to include your own IP2Location.io API key.
package com.example.ip2locationio; import com.google.gson.JsonObject; import com.ip2location.Configuration; import com.ip2location.IPGeolocation; import com.ip2location.DomainWhois; public class App { public static void main( String[] args ) throws Exception { // Configures IP2Location.io API key Configuration config = new Configuration(); String apiKey = "YOUR_API_KEY"; config.setApiKey(apiKey); IPGeolocation ipl = new IPGeolocation(config); // Lookup ip address geolocation data JsonObject myObj = ipl.Lookup("8.8.8.8", "en"); // the language parameter is only available for Plus and Security plans System.out.println(myObj); DomainWhois whois = new DomainWhois(config); // Lookup domain information JsonObject myObj2 = whois.Lookup("locaproxy.com"); System.out.println(myObj2); } }
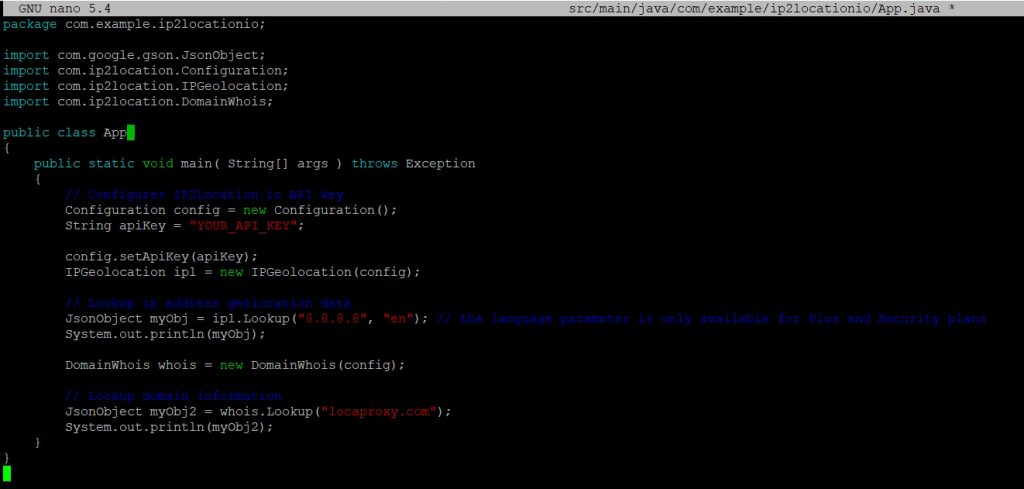
Testing the code
To compile the code, run the following command:
mvn clean package
To run the app, run the below command:
mvn exec:java -Dexec.mainClass="com.example.ip2locationio.App" -Dexec.cleanupDaemonThreads=false
You’ll see the results like below:
{"ip":"8.8.8.8","country_code":"US","country_name":"United States of America","region_name":"California","city_name":"Mountain View","latitude":37.405992,"longitude":-122.078515,"zip_code":"94043","time_zone":"-07:00","asn":"15169","as":"Google LLC","isp":"Google LLC","domain":"google.com","net_speed":"T1","idd_code":"1","area_code":"650","weather_station_code":"USCA0746","weather_station_name":"Mountain View","mcc":"-","mnc":"-","mobile_brand":"-","elevation":32,"usage_type":"DCH","address_type":"Anycast","continent":{"name":"North America","code":"NA","hemisphere":["north","west"],"translation":{"lang":"en","value":"North America"}},"district":"Santa Clara County","country":{"name":"United States of America","alpha3_code":"USA","numeric_code":840,"demonym":"Americans","flag":"https://cdn.ip2location.io/assets/img/flags/us.png","capital":"Washington, D.C.","total_area":9826675,"population":331002651,"currency":{"code":"USD","name":"United States Dollar","symbol":"$"},"language":{"code":"EN","name":"English"},"tld":"us","translation":{"lang":"en","value":"United States of America"}},"region":{"name":"California","code":"US-CA","translation":{"lang":"en","value":"California"}},"city":{"name":"Mountain View","translation":{"lang":"en","value":"Mountain View"}},"time_zone_info":{"olson":"America/Los_Angeles","current_time":"2023-06-07T20:10:10-07:00","gmt_offset":-25200,"is_dst":true,"sunrise":"05:47","sunset":"20:27"},"geotargeting":{"metro":"807"},"ads_category":"IAB19-11","ads_category_name":"Data Centers","is_proxy":false,"proxy":{"last_seen":7,"proxy_type":"DCH","threat":"-","provider":"-"}} {"domain":"locaproxy.com","domain_id":"1710914405_DOMAIN_COM-VRSN","status":"clientTransferProhibited https://icann.org/epp#clientTransferProhibited","create_date":"2012-04-03T02:34:32Z","update_date":"2021-12-03T02:54:57Z","expire_date":"2024-04-03T02:34:32Z","domain_age":4083,"whois_server":"whois.godaddy.com","registrar":{"iana_id":"146","name":"GoDaddy.com, LLC","url":"https://www.godaddy.com"},"registrant":{"name":"Registration Private","organization":"Domains By Proxy, LLC","street_address":"DomainsByProxy.com","city":"Tempe","region":"Arizona","zip_code":"85284","country":"US","phone":"+1.4806242599","fax":"+1.4806242598","email":"Select Contact Domain Holder link at https://www.godaddy.com/whois/results.aspx?domain=LOCAPROXY.COM"},"admin":{"name":"Registration Private","organization":"Domains By Proxy, LLC","street_address":"DomainsByProxy.com","city":"Tempe","region":"Arizona","zip_code":"85284","country":"US","phone":"+1.4806242599","fax":"+1.4806242598","email":"Select Contact Domain Holder link at https://www.godaddy.com/whois/results.aspx?domain=LOCAPROXY.COM"},"tech":{"name":"Registration Private","organization":"Domains By Proxy, LLC","street_address":"DomainsByProxy.com","city":"Tempe","region":"Arizona","zip_code":"85284","country":"US","phone":"+1.4806242599","fax":"+1.4806242598","email":"Select Contact Domain Holder link at https://www.godaddy.com/whois/results.aspx?domain=LOCAPROXY.COM"},"billing":{"name":"","organization":"","street_address":"","city":"","region":"","zip_code":"","country":"","phone":"","fax":"","email":""},"nameservers":["vera.ns.cloudflare.com","walt.ns.cloudflare.com"]}

Conclusion
It is that easy to integrate the IP2Location.io Java SDK in your Maven project. Hope you have found this article helpful in your journey to integrate IP2Location.io and IP2WHOIS into your applications.