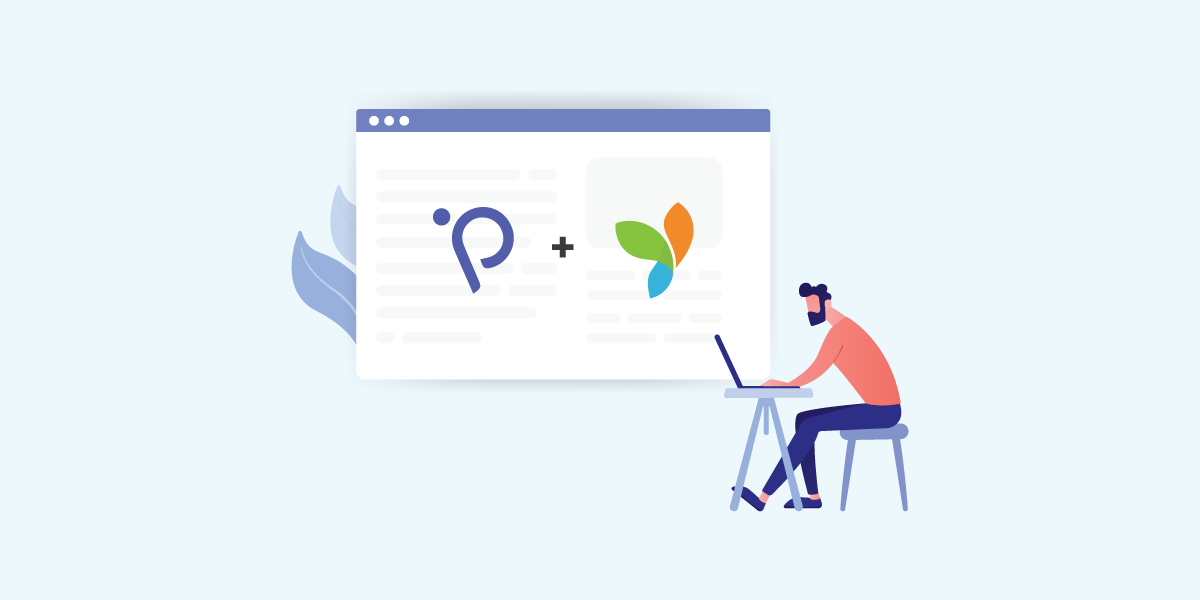
Yii is an open-source PHP web framework that aims to let the developers to create a website easily. It is based on the Model-View-Controller (MVC) pattern. It provides various components, for example database abstraction, caching, authentication, and security. With the aid of these components, developers can easily focus on the application logic. Also, Yii had a bunch of of extensions that can help developers to add certain functionality to the application.
Yii extensions are redistributable packages that can provide ready-to-use features to the application. Yii community often maintains and updates the extensions to make sure that the extensions are always compatible with the latest version of Yii. By default, Yii will install extensions that are uploaded to the Packagist repository. Yii encourages developers to use Composer to install the extension from Packagist.
In this tutorial, we will show you how to use IP2Location.io PHP SDK in Yii to retrieve the geolocation information for viewing and page redirection. IP2Location.io PHP SDK is a PHP package that allows users to retrieve a set of geolocation information for an IP address. The information includes country, region, district, city, latitude, longitude, ZIP code, time zone, ASN, ISP, domain, net speed, IDD code, area code, weather station data, MNC, MCC, mobile brand, elevation, usage type, address type, advertisement category and proxy data. The package supports both IPV4 and IPV6 lookup.
Before we get started, please make sure that you have properly installed and set up Yii and Composer. The IP2Location.io PHP SDK requires an API key to function. You may sign up for a free API key at https://www.ip2location.io/pricing. Do note that the continent, country, region and city translations are only available in the Plus and Security plans.
Displaying Geolocation Information
- First of all, if you have not installed the IP2Location.io PHP SDK, you can run the following command in the console to do so:
composer require ip2location/ip2location-io-php
- After that, create a template file called geolocation.php in your yii_project_root_directory/views/site/, and open the file in any text editor.
- Paste the following content into the file:
<?php use yii\helpers\Html; // Configures IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new \IP2LocationIO\IPGeolocation($config); ?> <p> This is a geolocation page. </p> <?php try { // Lookup IP address geolocation data $result = $ip2locationio->lookup('8.8.8.8'); echo 'IP Address : ' . $result->ip . "<br>"; echo 'Country Code : ' . $result->country_code . "<br>"; echo 'Country Name : ' . $result->country_name . "<br>"; echo 'Region Name : ' . $result->region_name . "<br>"; echo 'City Name : ' . $result->city_name . "<br>"; echo 'City Latitude : ' . $result->latitude . "<br>"; echo 'City Longitude : ' . $result->longitude . "<br>"; echo 'ZIP Code : ' . $result->zip_code . "<br>"; echo 'Time Zone : ' . $result->time_zone . "<br>"; echo 'ASN : ' . $result->asn . "<br>"; echo 'AS : ' . $result->as . "<br>"; echo 'Is Proxy : ' . (($result->is_proxy) ? 'TRUE' : 'FALSE') . "<br>"; echo 'ISP Name : ' . ($result->isp ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Domain Name : ' . ($result->domain ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Net Speed : ' . ($result->net_speend ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'IDD Code : ' . ($result->idd_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Area Code : ' . ($result->area_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Code : ' . ($result->weather_station_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Name : ' . ($result->weather_station_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MCC : ' . ($result->mcc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MNC : ' . ($result->mnc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Mobile Carrier : ' . ($result->mobile_brand ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Elevation : ' . ($result->elevation ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Usage Type : ' . ($result->usage_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Address Type : ' . ($result->address_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Code : ' . ($result->ads_category ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Name : ' . ($result->ads_category_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'District Name : ' . ($result->district ?? 'FIELD NOT SUPPORTED') . "<br>"; if (isset($result->continent)) { echo 'Continent Name : ' . $result->continent->name . "<br>"; echo 'Continent Code : ' . $result->continent->code . "<br>"; echo 'Continent Hemisphere : '; print_r($result->continent->hemisphere); echo "<br>"; echo 'Continent Translation : ' . $result->continent->translation->value . ' (' . $result->continent->translation->lang . ')' . "<br>"; } if (isset($result->country)) { echo 'Country Name : ' . $result->country->name . "<br>"; echo 'Country Alpha 3 Code : ' . $result->country->alpha3_code . "<br>"; echo 'Country Numeric Code : ' . $result->country->numeric_code . "<br>"; echo 'Country Demonym : ' . $result->country->demonym . "<br>"; echo 'Country Flag : ' . $result->country->flag . "<br>"; echo 'Country Capital : ' . $result->country->capital . "<br>"; echo 'Country Total Area : ' . $result->country->total_area . "<br>"; echo 'Country Population : ' . $result->country->population . "<br>"; echo 'Country Currency Code : ' . $result->country->currency->code . "<br>"; echo 'Country Currency Name : ' . $result->country->currency->name . ' (' . $result->country->currency->symbol . ')' . "<br>"; echo 'Country Language : ' . $result->country->language->name . ' (' . $result->country->language->code . ')' . "<br>"; echo 'Country TLD : ' . $result->country->tld . "<br>"; echo 'Country Translation : ' . $result->country->translation->value . ' (' . $result->country->translation->lang . ')' . "<br>"; } if (isset($result->region)) { echo 'Region Name : ' . $result->region->name . "<br>"; echo 'Region Code : ' . $result->region->code . "<br>"; echo 'Region Translation : ' . $result->region->translation->value . ' (' . $result->region->translation->lang . ')' . "<br>"; } if (isset($result->city)) { echo 'City Name : ' . $result->city->name . "<br>"; echo 'City Translation : ' . $result->city->translation->value . ' (' . $result->city->translation->lang . ')' . "<br>"; } if (isset($result->time_zone_info)) { echo 'Olson Time Zone : ' . $result->time_zone_info->olson . "<br>"; echo 'Current Time : ' . $result->time_zone_info->current_time . "<br>"; echo 'GMT Offset : ' . $result->time_zone_info->gmt_offset . "<br>"; echo 'Is DST : '; echo ($result->time_zone_info->is_dst) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'Sunrise Time : ' . $result->time_zone_info->sunrise . "<br>"; echo 'Sunset Time : ' . $result->time_zone_info->sunset . "<br>"; } if (isset($result->geotargeting)) { echo 'Metro Code : ' . $result->geotargeting->metro . "<br>"; } if (isset($result->proxy)) { echo 'Proxy Last Seen : ' . $result->proxy->last_seen . "<br>"; echo 'Proxy Type : ' . $result->proxy->proxy_type . "<br>"; echo 'Proxy Threat : ' . $result->proxy->threat . "<br>"; echo 'Proxy Provider : ' . $result->proxy->provider . "<br>"; } } catch(Exception $e) { echo $e->getCode() . ": " . $e->getMessage(); } ?>
- Next, open the SiteController.php file located at your yii_project_root_directory/controllers/ in any text editor, and paste the following contents inside the SiteController class:
public function actionGeolocation()
{
return $this->render(‘geolocation’);
}
- Enter the URL /index.php?r=site%2Fgeolocation and run. You should be able to see the information of 8.8.8.8 IP address.
Redirecting user based on its geolocation
- First of all, if you have not installed the IP2Location.io PHP SDK, you can run the following command in the console to do so:
composer require ip2location/ip2location-io-php
- Add the below lines into the Controller file that you want to use for redirection (Note that you can change the function name to another name with the action as the prefix):
public function actionRedirect() { $ip = Yii::$app->request->userIP; // Configure IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new \IP2LocationIO\IPGeolocation($config); // Get visitor IP address for lookup $result = $ip2locationio->lookup($ip); if ($result->country_code == 'US') { return $this->render('index'); } }
After following this tutorial, you should be able to perform geolocation lookup and redirection by using IP2Location.io PHP SDK in Yii. You can make use the geolocation information in various area, for example providing region-customization contents.
Learn more on how to use IP2Location.io PHP SDK in various frameworks.