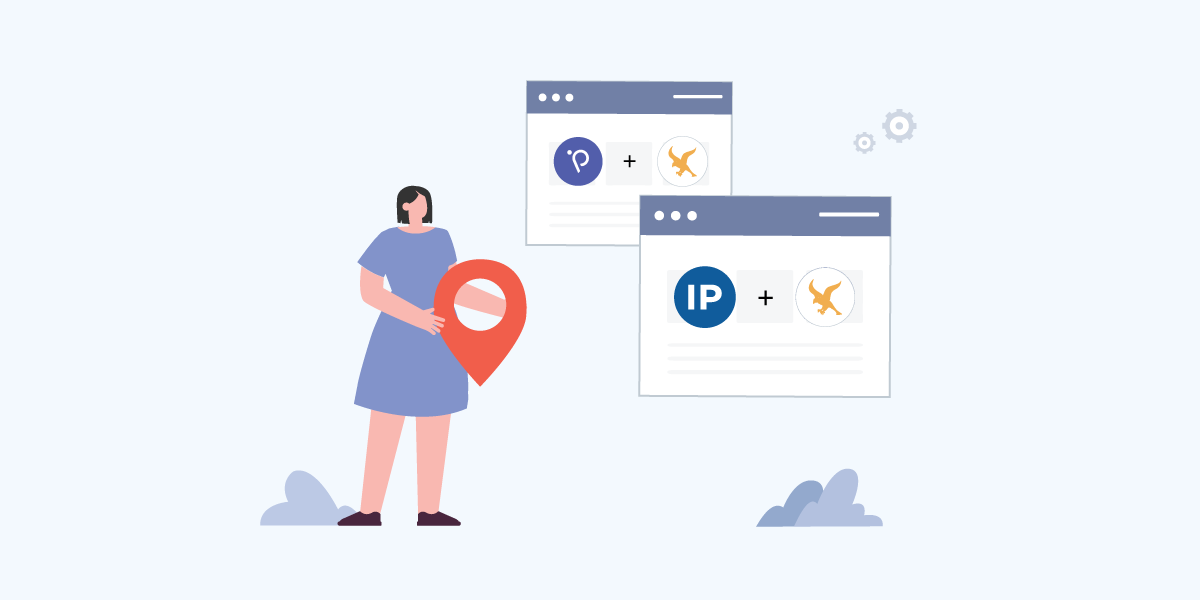
Falcon is a Python web framework that is weightless and efficient. Falcon was designed in such a way that it is simple yet powerful. It supports the Web Server Gateway Interface (WSGI) and Asynchronous Server Gateway Interface (ASGI) protocols. It provides all necessary features to build a web application, such as routing and middleware components.
Besides that, their codebase is also extensible. Users are free to use any community-developed add-ons and packages on their application. Falcon encourages the community to develop Falcon add-ons and packages. These add-ons and packages can be found in PyPI. To install these add-ons, user can use Pip which is a package installer for Python.
IP2Location and IP2Location.io Python SDK
In this tutorial, we are going to guide you on how to use IP2Location and IP2Location.io Python SDK in Falcon to retrieve and display geolocation information. IP2Location Python SDK is a Python package that enables user to search for a bunch of geolocation data based on an IP address. For example, country, region, district, city, latitude, longitude, ZIP code, time zone, ISP, domain name, connection speed, IDD code, area code, weather station code, weather station name, MNC, MCC, mobile brand, elevation, usage type, address type, IAB category and ASN.
Meanwhile, IP2Location.io Python SDK is a Python package that allows users to retrieve a set of geolocation information for an IP address. The information includes country, region, district, city, latitude, longitude, ZIP code, time zone, ASN, ISP, domain, net speed, IDD code, area code, weather station data, MNC, MCC, mobile brand, elevation, usage type, address type, advertisement category and proxy data. Both IP2Location and IP2Location.io Python SDK support IPv4 and IPv6 lookup.
Getting Started
Before we get started, please make sure that you have properly installed and set up Falcon and Pip. You will also need to install a WSGI server. You can install Gunicorn for Linux or Waitress for Windows. The IP2Location Python SDK requires the BIN database, however you can obtain a free database from https://lite.ip2location.com/. The IP2Location.io Python SDK requires an API key to function. You may sign up for a free API key at https://www.ip2location.io/pricing. Do note that the continent, country, region and city translations are only available in the Plus and Security plans.
Display Geolocation Data Using IP2Location Python SDK
- First of all, if you have not installed the IP2Location Python SDK, you can run the following command in the console to do so:
pip install IP2Location
- In your Falcon project root, create a new directory called look, and inside the look directory, create a new file called geolocations.py.
- Open the file in any text editor, and paste the following code into the file:
import IP2Location import falcon class Resource: def on_get(self, req, resp): database = IP2Location.IP2Location("path/to/your/IP2LOCATION_BIN_DATABASE.BIN") rec = database.get_all("8.8.8.8") html_response = "Country Code : " + rec.country_short + '\n' html_response = html_response + "Country Name : " + rec.country_long + '\n' html_response = html_response + 'Region Name : ' + rec.region + '\n' html_response = html_response + 'City Name : ' + rec.city + '\n' html_response = html_response + 'ISP Name : ' + rec.isp + '\n' html_response = html_response + 'Latitude : ' + rec.latitude + '\n' html_response = html_response + 'Longitude : ' + rec.longitude + '\n' html_response = html_response + 'Domain Name : ' + rec.domain + '\n' html_response = html_response + 'ZIP Code : ' + rec.zipcode + '\n' html_response = html_response + 'Time Zone : ' + rec.timezone + '\n' html_response = html_response + 'Net Speed : ' + rec.idd_code + '\n' html_response = html_response + 'Area Code : ' + rec.area_code + '\n' html_response = html_response + 'IDD Code : ' + rec.idd_code + '\n' html_response = html_response + 'Weather Station Code : ' + rec.weather_code + '\n' html_response = html_response + 'Weather Station Name : ' + rec.weather_name + '\n' html_response = html_response + 'MCC : ' + rec.mcc + '\n' html_response = html_response + 'MNC : ' + rec.mnc + '\n' html_response = html_response + 'Mobile Carrier : ' + rec.mobile_brand + '\n' html_response = html_response + 'Elevation : ' + rec.elevation + '\n' html_response = html_response + 'Usage Type : ' + rec.usage_type + '\n' html_response = html_response + 'Address Type : ' + rec.address_type + '\n' html_response = html_response + 'Category : ' + rec.category + '\n' html_response = html_response + 'District : ' + rec.district + '\n' html_response = html_response + 'ASN : ' + rec.asn + '\n' html_response = html_response + 'AS : ' + rec.as_name + '\n' resp.text = html_response # The following line can be omitted because 200 is the default # status returned by the framework, but it is included here to # illustrate how this may be overridden as needed. resp.status = falcon.HTTP_200
- In the same look directory, create another file called app.py.
- Open the file in any text editor, and paste the following code into the file:
import falcon from .geolocations import Resource app = application = falcon.App() geolocations = Resource() app.add_route('/geolocation', geolocations)
- In your command prompt, cd to your Falcon project root directory. To start a local WSGI server, you can run this command
gunicorn --reload look.app
for Linux or this commandwaitress-serve --port=8000 look.app:app
for Windows. - You can now see the page by accessing to this URL http://localhost:8000/geolocation in your browser.
Display Geolocation Data Using IP2Location.io Python SDK
- First of all, if you have not installed the IP2Location.io Python SDK, you can run the following command in the console to do so:
pip install IP2Location-io
- In your Falcon project root, create a new directory called look, and inside the look directory, create a new file called geolocations.py.
- Open the file in any text editor, and paste the following code into the file:
import ip2locationio import falcon class Resource: def on_get(self, req, resp): # Configures IP2Location.io API key configuration = ip2locationio.Configuration('YOUR_API_KEY') ipgeolocation = ip2locationio.IPGeolocation(configuration) result = ipgeolocation.lookup('8.8.8.8') html_response = 'IP Address : ' + result['ip'] + '\n' html_response = html_response + 'Country Code : ' + result['country_code'] + '\n' html_response = html_response + 'Country Name : ' + result['country_name'] + '\n' html_response = html_response + 'Region Name : ' + result['region_name'] + '\n' html_response = html_response + 'City Name : ' + result['city_name'] + '\n' html_response = html_response + 'City Latitude : ' + str(result['latitude']) + '\n' html_response = html_response + 'City Longitude : ' + str(result['longitude']) + '\n' html_response = html_response + 'ZIP Code : ' + result['zip_code'] + '\n' html_response = html_response + 'Time Zone : ' + result['time_zone'] + '\n' html_response = html_response + 'ASN : ' + result['asn'] + '\n' html_response = html_response + 'AS : ' + result['as'] + '\n' if result['is_proxy']: html_response = html_response + 'Is Proxy : TRUE\n' else: html_response = html_response + 'Is Proxy : FALSE\n' keys = {'isp': 'ISP Name ', 'domain': 'Domain Name ', 'domain': 'Domain Name ', 'net_speed': 'Net Speed ', 'idd_code': 'IDD Code ', 'area_code': 'Area Code ', 'weather_station_code': 'Weather Station Code : ', 'weather_station_name': 'Weather Station Name ', 'mcc': 'MCC ', 'mnc': 'MNC ', 'mobile_brand': 'Mobile Carrier ', 'elevation': 'Elevation ', 'usage_type': 'Usage Type ', 'address_type': 'Address Type ', 'ads_category': 'Category Code ', 'ads_category_name': 'Category Name ', 'district': 'District Name '} for key in keys: if result[key]: html_response = html_response + keys[key] + ': ' + str(result[key]) + '\n' if result['continent']: html_response = html_response + 'Continent Name : ' + result['continent']['name'] + '\n' html_response = html_response + 'Continent Code : ' + result['continent']['code'] + '\n' html_response = html_response + 'Continent Hemisphere : ' + ', '.join(result['continent']['hemisphere']) + '\n' html_response = html_response + 'Continent Translation : ' + str(result['continent']['translation']['value']) + ' (' + str(result['continent']['translation']['lang']) + ')\n' if result['country']: html_response = html_response + 'Country Name : ' + result['country']['name'] + '\n' html_response = html_response + 'Country Alpha 3 Code : ' + result['country']['alpha3_code'] + '\n' html_response = html_response + 'Country Numeric Code : ' + str(result['country']['numeric_code']) + '\n' html_response = html_response + 'Country Demonym : ' + result['country']['demonym'] + '\n' html_response = html_response + 'Country Flag : ' + result['country']['flag'] + '\n' html_response = html_response + 'Country Capital : ' + result['country']['capital'] + '\n' html_response = html_response + 'Country Total Area : ' + str(result['country']['total_area']) + '\n' html_response = html_response + 'Country Population : ' + str(result['country']['population']) + '\n' html_response = html_response + 'Country Currency Code : ' + result['country']['currency']['code'] + '\n' html_response = html_response + 'Country Currency Name : ' + str(result['country']['currency']['name']) + ' (' + str(result['country']['currency']['symbol']) + ')\n' html_response = html_response + 'Country Language : ' + str(result['country']['language']['name']) + ' (' + str(result['country']['language']['code']) + ')\n' html_response = html_response + 'Country TLD : ' + result['country']['tld'] + '\n' html_response = html_response + 'Country Translation : ' + str(result['country']['translation']['value']) + ' (' + str(result['country']['translation']['lang']) + ')\n' if result['region']: html_response = html_response + 'Region Name : ' + result['region']['name'] + '\n' html_response = html_response + 'Region Code : ' + result['region']['code'] + '\n' html_response = html_response + 'Region Translation : ' + str(result['region']['translation']['value']) + ' (' + str(result['region']['translation']['lang']) + ')\n' if result['city']: html_response = html_response + 'City Name : ' + result['city']['name'] + '\n' html_response = html_response + 'City Translation : ' + str(result['city']['translation']['value']) + ' (' + str(result['city']['translation']['lang']) + ')\n' if result['time_zone_info']: html_response = html_response + 'Olson Time Zone : ' + result['time_zone_info']['olson'] + '\n' html_response = html_response + 'Current Time : ' + result['time_zone_info']['current_time'] + '\n' html_response = html_response + 'GMT Offset : ' + str(result['time_zone_info']['gmt_offset']) + '\n' if result['time_zone_info']['is_dst']: html_response = html_response + 'Is DST : TRUE\n' else: html_response = html_response + 'Is DST : FALSE\n' html_response = html_response + 'Sunrise Time : ' + result['time_zone_info']['sunrise'] + '\n' html_response = html_response + 'Sunset Time : ' + result['time_zone_info']['sunset'] + '\n' if result['geotargeting']: html_response = html_response + 'Metro Code : ' + result['geotargeting']['metro'] + '\n' if result['proxy']: html_response = html_response + 'Proxy Last Seen : ' + str(result['proxy']['last_seen']) + '\n' html_response = html_response + 'Proxy Type : ' + result['proxy']['proxy_type'] + '\n' html_response = html_response + 'Proxy Threat : ' + result['proxy']['threat'] + '\n' html_response = html_response + 'Proxy Provider : ' + result['proxy']['provider'] + '\n' resp.text = html_response # The following line can be omitted because 200 is the default # status returned by the framework, but it is included here to # illustrate how this may be overridden as needed. resp.status = falcon.HTTP_200
- In the same look directory, create another file called app.py.
- Open the file in any text editor, and paste the following code into the file:
import falcon from .geolocations import Resource app = application = falcon.App() geolocations = Resource() app.add_route('/geolocation', geolocations)
- In your command prompt, cd to your Falcon project root directory. To start a local WSGI server, you can run this command
gunicorn --reload look.app
for Linux or this commandwaitress-serve --port=8000 look.app:app
for Windows. - You can now see the page by accessing to this URL http://localhost:8000/geolocation in your browser.
After following this tutorial, you should able to utilize IP2Location and IP2Location.io Python SDK in your web application to perform IP geolocation lookup. The geolocation information can extend your web application functionality, such as serving personalized contents to your user or identifying your customer’s origin.