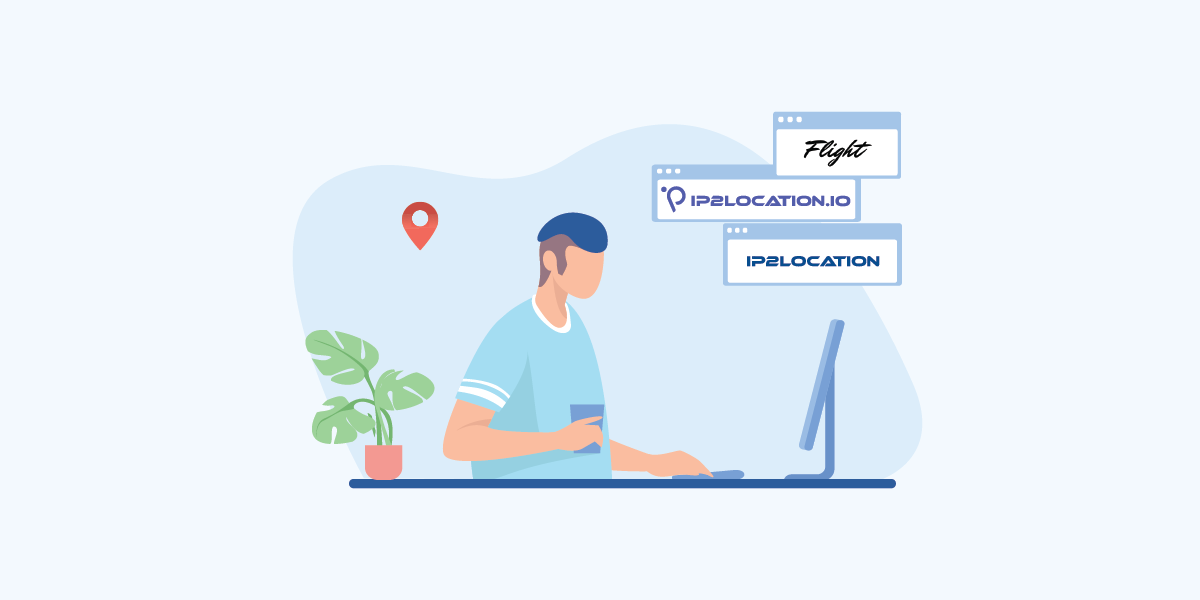
The Flight framework is a lightweight and fast PHP micro-framework that allows developers to easily build web applications with a minimal footprint and a straightforward routing system.
Flight PHP packages, available on Packagist repository, are reusable code modules that can be effortlessly installed using Composer. These packages empower Flight applications with enhanced functionality and easy customization options.
IP2Location and IP2Location.io PHP SDKs
The IP2Location PHP package is a specialized software library that enables PHP developers to obtain geolocation information from IP addresses.
The IP2Location PHP package employs the extensive IP2Location BIN database to offer an extensive array of geographic details of IP addresses. It including country, region, district, city, latitude, longitude, ZIP code, time zone, ISP, domain name, connection speed, IDD code, area code, weather station code, weather station name, MNC, MCC, mobile brand, elevation, usage type, address type, IAB category and ASN. It supports both IPv4 and IPv6 address lookups, it is well-suited for diverse PHP projects.
Conversely, the IP2Location.io PHP SDK streamlines the incorporation of the IP2Location.io geolocation service into PHP applications. It facilitates seamless retrieval of geolocation details using a RESTful API that operates on IP addresses.
With the PHP SDK, developers can seamlessly integrate IP geolocation features into their applications. They can easily obtain details like country, region, district, city, latitude, longitude, ZIP code, time zone, ASN, ISP, domain, net speed, IDD code, area code, weather station data, MNC, MCC, mobile brand, elevation, usage type, address type, advertisement category and proxy data. The SDK eliminates the need for manual HTTP requests and supports both IPv4 and IPv6 address lookups.
Getting Started
This tutorial will demonstrate how to effectively utilize the IP2Location and IP2Location.io PHP SDKs with the Flight framework to retrieve geolocation information for the purpose of displaying it.
To begin with this tutorial, ensure that the Flight framework is correctly configured. For the IP2Location PHP SDK, a BIN database is required, which can be obtained for free from https://lite.ip2location.com/. The IP2Location.io PHP SDK relies on an API key, accessible through a free account registration at https://www.ip2location.io/pricing. However, please be aware that continent, country, region, and city translations are only available in the Plus and Security Plans.
Display Geolocation Data Using IP2Location PHP SDK
- To obtain the package and incorporate it into the Flight platform, use the following command.
composer require ip2location/ip2location-php
- In any text editor, access the index.php file.
- Add the following codes into the index.php file for utilizing the IP2Location PHP SDK and showcasing geolocation information.
<?php //This is used to load the required classes and dependencies require 'vendor/autoload.php'; //Define a route for the root URL Flight::route('/', function () { //Replace with the IP address you want to geolocate $ipAddress = "8.8.8.8"; //Specify the path to the BIN database file $database = new \IP2Location\Database('path/to/your/IP2Location_BIN_DATABASE.BIN', \IP2Location\Database::FILE_IO); //Retrieve geolocation data $record = $database->lookup($ipAddress, \IP2Location\Database::ALL); //This is to display all the geolocation data echo '<pre>'; echo 'IP Number : ' . $record['ipNumber'] . "\n"; echo 'IP Version : ' . $record['ipVersion'] . "\n"; echo 'IP Address : ' . $record['ipAddress'] . "\n"; echo 'Country Code : ' . $record['countryCode'] . "\n"; echo 'Country Name : ' . $record['countryName'] . "\n"; echo 'Region Name : ' . $record['regionName'] . "\n"; echo 'City Name : ' . $record['cityName'] . "\n"; echo 'Latitude : ' . $record['latitude'] . "\n"; echo 'Longitude : ' . $record['longitude'] . "\n"; echo 'Area Code : ' . $record['areaCode'] . "\n"; echo 'IDD Code : ' . $record['iddCode'] . "\n"; echo 'Weather Station Code : ' . $record['weatherStationCode'] . "\n"; echo 'Weather Station Name : ' . $record['weatherStationName'] . "\n"; echo 'MCC : ' . $record['mcc'] . "\n"; echo 'MNC : ' . $record['mnc'] . "\n"; echo 'Mobile Carrier : ' . $record['mobileCarrierName'] . "\n"; echo 'Usage Type : ' . $record['usageType'] . "\n"; echo 'Elevation : ' . $record['elevation'] . "\n"; echo 'Net Speed : ' . $record['netSpeed'] . "\n"; echo 'Time Zone : ' . $record['timeZone'] . "\n"; echo 'ZIP Code : ' . $record['zipCode'] . "\n"; echo 'Domain Name : ' . $record['domainName'] . "\n"; echo 'ISP Name : ' . $record['isp'] . "\n"; echo 'Address Type : ' . $record['addressType'] . "\n"; echo 'Category : ' . $record['category'] . "\n"; echo 'District : ' . $record['district'] . "\n"; echo 'ASN : ' . $record['asn'] . "\n"; echo 'AS : ' . $record['as'] . "\n"; echo '</pre>'; }); //Initiates the execution of the "Flight" function Flight::start(); ?>
- Initiate a web server by executing the following command in the CLI from your project directory:
php -S localhost:8000
- Now you can view the Flight application by opening your browser and visiting “http://localhost:8000”. The webpage will show the IP geolocation information obtained from the IP2Location BIN database.
- Done.
Display Geolocation Data Using IP2Location.io PHP SDK
- To obtain the package and incorporate it into the Flight framework, use the following command.
composer require ip2location/ip2location-io-php
- In any text editor, access the index.php file.
- Add the following codes into the index.php file for utilizing the IP2Location.io PHP SDK and showcasing geolocation information.
<?php //This is used to load the required classes and dependencies require 'vendor/autoload.php'; //Define a route for the root URL Flight::route('/', function () { //Replace with your actual API key $apiKey = "YOUR_API_KEY"; //Replace with the IP address you want to geolocate $ipAddress = "8.8.8.8"; //This class represents the configuration for the IP2Location.io API $config =new \IP2LocationIO\Configuration($apiKey); //This class is used to perform the geolocation lookup $ip2locationio = new \IP2LocationIO\IPGeolocation($config); //Sends a request to the IP2Location.io API to retrieve the geolocation information $response = $ip2locationio->lookup($ipAddress); try{ echo '<pre>'; echo 'IP Address : ' . $response->ip . "<br>"; echo 'Country Code : ' . $response->country_code . "<br>"; echo 'Country Name : ' . $response->country_name . "<br>"; echo 'Region Name : ' . $response->region_name . "<br>"; echo 'City Name : ' . $response->city_name . "<br>"; echo 'City Latitude : ' . $response->latitude . "<br>"; echo 'City Longitude : ' . $response->longitude . "<br>"; echo 'ZIP Code : ' . $response->zip_code . "<br>"; echo 'Time Zone : ' . $response->time_zone . "<br>"; echo 'ASN : ' . $response->asn . "<br>"; echo 'AS : ' . $response->as . "<br>"; echo 'Is Proxy : '; echo ($response->is_proxy) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'ISP Name : ' . ($response->isp ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Domain Name : ' . ($response->domain ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Net Speed : ' . ($response->net_speend ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'IDD Code : ' . ($response->idd_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Area Code : ' . ($response->area_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Code : ' . ($response->weather_station_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Name : ' . ($response->weather_station_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MCC : ' . ($response->mcc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MNC : ' . ($response->mnc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Mobile Carrier : ' . ($response->mobile_brand ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Elevation : ' . ($response->elevation ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Usage Type : ' . ($response->usage_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Address Type : ' . ($response->address_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Code : ' . ($response->ads_category ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Name : ' . ($response->ads_category_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'District Name : ' . ($response->district ?? 'FIELD NOT SUPPORTED') . "<br>"; if (isset($response->continent)) { echo 'Continent Name : ' . $response->continent->name . "<br>"; echo 'Continent Code : ' . $response->continent->code . "<br>"; echo 'Continent Hemisphere : '; print_r($response->continent->hemisphere); echo "<br>"; echo 'Continent Translation : ' . $response->continent->translation->value . ' (' . $response->continent->translation->lang . ')' . "<br>"; } if (isset($response->country)) { echo 'Country Name : ' . $response->country->name . "<br>"; echo 'Country Alpha 3 Code : ' . $response->country->alpha3_code . "<br>"; echo 'Country Numeric Code : ' . $response->country->numeric_code . "<br>"; echo 'Country Demonym : ' . $response->country->demonym . "<br>"; echo 'Country Flag : ' . $response->country->flag . "<br>"; echo 'Country Capital : ' . $response->country->capital . "<br>"; echo 'Country Total Area : ' . $response->country->total_area . "<br>"; echo 'Country Population : ' . $response->country->population . "<br>"; echo 'Country Currency Code : ' . $response->country->currency->code . "<br>"; echo 'Country Currency Name : ' . $response->country->currency->name . ' (' . $response->country->currency->symbol . ')' . "<br>"; echo 'Country Language : ' . $response->country->language->name . ' (' . $response->country->language->code . ')' . "<br>"; echo 'Country TLD : ' . $response->country->tld . "<br>"; echo 'Country Translation : ' . $response->country->translation->value . ' (' . $response->country->translation->lang . ')' . "<br>"; } if (isset($response->region)) { echo 'Region Name : ' . $response->region->name . "<br>"; echo 'Region Code : ' . $response->region->code . "<br>"; echo 'Region Translation : ' . $response->region->translation->value . ' (' . $response->region->translation->lang . ')' . "<br>"; } if (isset($response->city)) { echo 'City Name : ' . $response->city->name . "<br>"; echo 'City Translation : ' . $response->city->translation->value . ' (' . $response->city->translation->lang . ')' . "<br>"; } if (isset($response->time_zone_info)) { echo 'Olson Time Zone : ' . $response->time_zone_info->olson . "<br>"; echo 'Current Time : ' . $response->time_zone_info->current_time . "<br>"; echo 'GMT Offset : ' . $response->time_zone_info->gmt_offset . "<br>"; echo 'Is DST : '; echo ($response->time_zone_info->is_dst) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'Sunrise Time : ' . $response->time_zone_info->sunrise . "<br>"; echo 'Sunset Time : ' . $response->time_zone_info->sunset . "<br>"; } if (isset($response->geotargeting)) { echo 'Metro Code : ' . $response->geotargeting->metro . "<br>"; } if (isset($response->proxy)) { echo 'Proxy Last Seen : ' . $response->proxy->last_seen . "<br>"; echo 'Proxy Type : ' . $response->proxy->proxy_type . "<br>"; echo 'Proxy Threat : ' . $response->proxy->threat . "<br>"; echo 'Proxy Provider : ' . $response->proxy->provider . "<br>"; } echo '</pre>'; }catch(Exception $e){ echo "Error: ". $e->getMessage(); } }); //Initiates the execution of the "Flight" function Flight::start(); ?>
- Initiate a web server by executing the following command in the CLI from your project directory:
php -S localhost:8000
- Now you can view the Flight application by opening your browser and visiting “http://localhost:8000”. The webpage will show the IP geolocation info obtained from the IP2Location.io API.
- Done.
At the end of this tutorial, you will gain the ability to perform an IP geolocation lookup within the Flight framework, using the IP2Location and IP2Location.io PHP SDKs. This skill will allow you to retrieve geolocation data, aiding in user identification and data analysis.
Learn more on how to use IP2Location.io PHP SDK in various frameworks.