Introduction
In this tutorial, we will demonstrate how to use the image sprite that consists of 249 country flags for website display. In a nutshell, it is a method that combines, usually, multiple small images into a single image file for usage.
Image sprite and its advantages
What are the advantages of doing so? To elaborate, when the website needs to display one image, it will make one HTTP/HTTPS request to the server for the image file retrieval. If the website needs to display 20 images, then the website will have to make 20 round trips for the complete display. These round trips are time-consuming. However, by combining 20 images into a single image sprite, the website will now just need to make a single HTTP/HTTPS request for the same purpose. Inevitably, this will greatly reduce the page loading time, by cutting down the excessive HTTP/HTTPS round trips to only one. Imagine that if you were going to get those images across continents, how much time you could have saved with the image sprite.
Anyway, the image sprite is useful if you are going to display multiple images on a web page, if you only need a single image, an individual image file loading is the more feasible approach. Regarding the set of images provided by IP2Location, we provide both individual image files and also the image sprite, so that you can decide which one works best for you.
Getting Started – Using image sprite
- Download the country flags zip file. There are 7 types of country flag designs available according to the different sizes of the country flag, which are 16×16, 32×32, and 64×64.
- Then, unzip the file and copy the “image_sprite” folder to your project directory.
- In the header tag of your page, call the image sprite CSS file like this:
<link href="image_sprite/ip2location-image-sprite.css" rel="stylesheet">
- Pick the flag design and size, then display it by using the particular class name. For instance, to display a Vietnam country flag in pole design with a 64px size from the image sprite, you will need to use the code below:
<span class="ip2location-flag-64 flag-pole flag-vn"></span>
These classes represents different usages. Theflag-pole
class indicates the flag design whereas theip2location-flag-64
andflag-vn
classes indicate the country flag for 64px size.
For other flag design display, you can refer to classes below:
Square design:flag-square
Square rounded design:flag-square-rounded
Square bended design:flag-square-bended
Square shadow design:flag-square-shadow
Round design:flag-round
Pole design:flag-pole
Pin design:flag-pin
- Lastly, save the changes made and refresh the website in your browser to see the changes.
Example of using image sprite
Source codes
Below are the complete source codes for the country flags display. You can copy the codes and use it in your project.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <title>Image Sprite</title> <!-- Bootstrap core CSS --> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css" integrity="sha384-ggOyR0iXCbMQv3Xipma34MD+dH/1fQ784/j6cY/iJTQUOhcWr7x9JvoRxT2MZw1T" crossorigin="anonymous"> <!-- Image Sprite CSS --> <link href="image_sprite/ip2location-image-sprite.css" rel="stylesheet"> </head> <body> <!-- Navigation --> <nav class="navbar navbar-expand-lg navbar-dark bg-dark static-top"> <div class="container"> <a class="navbar-brand" href="#">Country Flag Usage Example</a> </div> </nav> <!-- Page Content --> <div class="container"> <div class="row"> <div class="col-md-12 text-center"> <table class="table table-bordered mt-5"> <tbody> <tr> <th></th> <th style="width:15%">Square</th> <th style="width:15%">Square Rounded</th> <th style="width:15%">Square Bended</th> <th style="width:15%">Square Shadow</th> <th style="width:15%">Round</th> <th style="width:15%">Pole</th> <th style="width:15%">Pin</th> </tr> <tr> <th>16px</th> <td class="align-middle"> <div><span class="ip2location-flag-16 flag-square flag-us"></span></div> <div>United States</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-16 flag-square-rounded flag-de"></span></div> <div>Germany</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-16 flag-square-bended flag-my"></span></div> <div>Malaysia</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-16 flag-square-shadow flag-gb"></span></div> <div>United Kingdom</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-16 flag-round flag-au"></span></div> <div>Australia</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-16 flag-pole flag-vn"></span></div> <div>Vietnam</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-16 flag-pin flag-in"></span></div> <div>India</div> </td> </tr> <tr> <th>32px</th> <td class="align-middle"> <div><span class="ip2location-flag-32 flag-square flag-us"></span></div> <div>United States</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-32 flag-square-rounded flag-de"></span></div> <div>Germany</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-32 flag-square-bended flag-my"></span></div> <div>Malaysia</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-32 flag-square-shadow flag-gb"></span></div> <div>United Kingdom</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-32 flag-round flag-au"></span></div> <div>Australia</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-32 flag-pole flag-vn"></span></div> <div>Vietnam</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-32 flag-pin flag-in"></span></div> <div>India</div> </td> </tr> <tr> <th>64px</th> <td class="align-middle"> <div><span class="ip2location-flag-64 flag-square flag-us"></span></div> <div>United States</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-64 flag-square-rounded flag-de"></span></div> <div>Germany</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-64 flag-square-bended flag-my"></span></div> <div>Malaysia</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-64 flag-square-shadow flag-gb"></span></div> <div>United Kingdom</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-64 flag-round flag-au"></span></div> <div>Australia</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-64 flag-pole flag-vn"></span></div> <div>Vietnam</div> </td> <td class="align-middle"> <div><span class="ip2location-flag-64 flag-pin flag-in"></span></div> <div>India</div> </td> </tr> </tbody> </table> </div> </div> </div> <script src="https://code.jquery.com/jquery-3.3.1.slim.min.js" integrity="sha384-q8i/X+965DzO0rT7abK41JStQIAqVgRVzpbzo5smXKp4YfRvH+8abtTE1Pi6jizo" crossorigin="anonymous"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js" integrity="sha384-UO2eT0CpHqdSJQ6hJty5KVphtPhzWj9WO1clHTMGa3JDZwrnQq4sF86dIHNDz0W1" crossorigin="anonymous"></script> <script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js" integrity="sha384-JjSmVgyd0p3pXB1rRibZUAYoIIy6OrQ6VrjIEaFf/nJGzIxFDsf4x0xIM+B07jRM" crossorigin="anonymous"></script> </body> </html>
Output
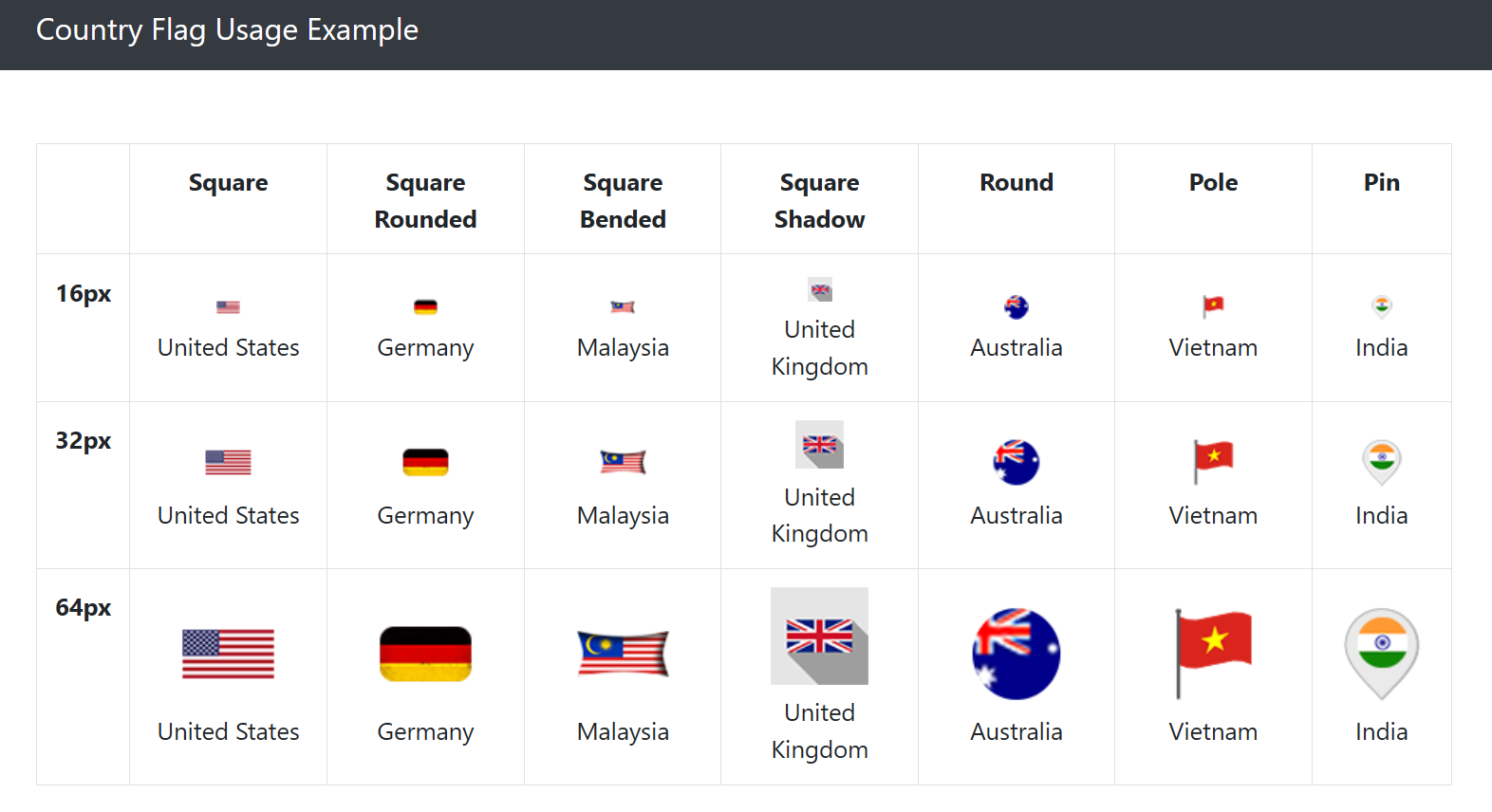
Video
IP2Location Country Flags
Display multiple of country flags in your website by using the image sprite.