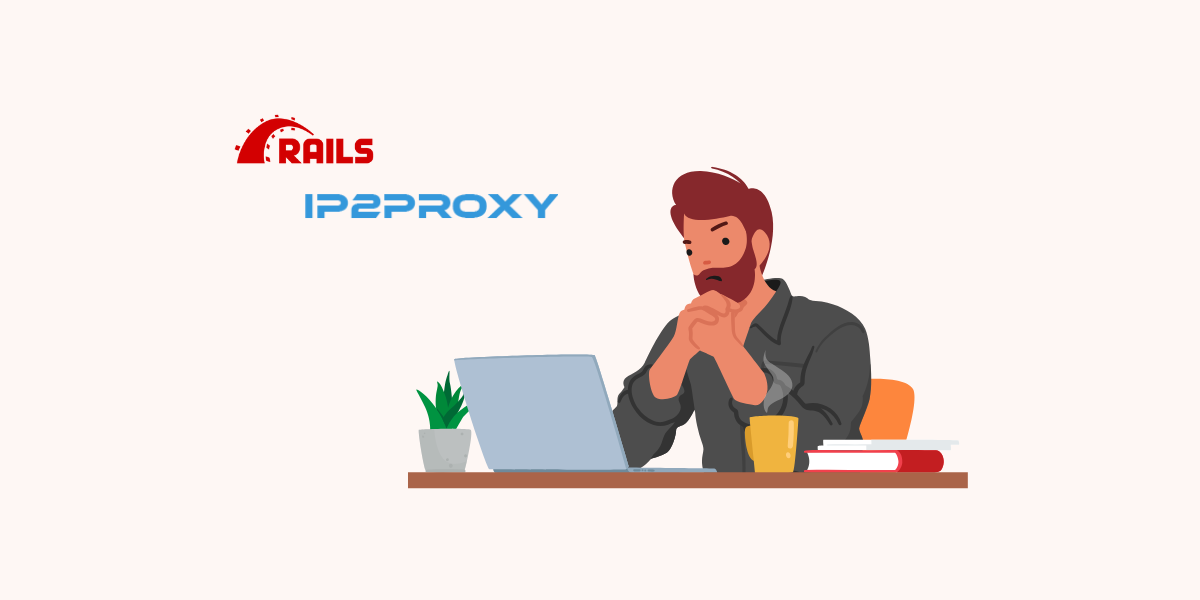
Ruby on Rails, commonly known as Rails, is an open-source web application framework built using the Ruby programming language. It adheres to the Model-View-Controller (MVC) architectural pattern, a design standard that divides an application’s structure into three distinct components: models, views and controllers. Models handle data and business logic, views manage the user interface and controllers orchestrate the flow between models and views. This separation of concerns makes Rails applications organized and maintainable. One of Rails’ key principles is “convention over configuration,” which means it provides pre-set conventions to streamline the development process, reducing the need for extensive manual setup.
In the Rails framework, reusable code modules are packaged into what are known as “gems.” These gems function as libraries that extend or enhance the capabilities of a Rails application. They provide a wide range of functionalities, from authentication systems and payment processing to API integrations and database management. Gems can be easily included in a Rails project by adding them to the Gemfile and are managed using Bundler, a dependency management tool. This modular approach allows developers to quickly incorporate powerful features without writing code from scratch, accelerating the development process and maintaining a clean, modular structure within the application.
IP2Proxy Ruby on Rails library, a Rails library that allows user to reverse search of IP address to detect VPN servers, open proxies, web proxies, TOR exit nodes, search engine robots, data center ranges, residential proxies, consumer privacy networks, and enterprise private networks using IP2Proxy BIN database. Other information available includes proxy type, country, state, city, ISP, domain name, usage type, AS number, AS name, threats, last seen date and provider names. It lookup the proxy IP address from IP2Proxy BIN Data file.
This tutorial demonstrates how to utilize the IP2Proxy library in a Ruby on Rails application to retrieve proxy-related information. Before proceeding, it’s assumed that the Ruby on Rails framework has already been correctly configured and set up.
Display Proxy Data Sample Code
- Add this lines to your Ruby on Rails server Gemfile.
gem 'ip2proxy_ruby'
gem 'ip2proxy_rails'
- Execute it by running the command.
$ bundle install
- Open the preferred file in the config/environments directory. Add the following code to the chosen configuration file after the Rails.application.configure do line.
config.ip2proxy_db_path = Rails.root.join('lib', 'assets', 'ip2proxy_rails', 'IP2PROXY.BIN').to_s
- Download IP2Proxy BIN database from https://www.ip2location.com/proxy-database
- Create a folder named as ip2proxy_rails in the lib/assets directory.
- Unzip and copy the BIN file into lib/assets/ ip2proxy_rails folder.
- Rename the BIN file to IP2PROXY.BIN.
- Create a TestController using the below command line
bin/rails generate controller Test index --skip-routes
- Open the app/controllers/test_controller.rb in any text editor.
- Add the below lines into the controller file.
require 'ip2proxy_rails' class TestController < ApplicationController def index proxy_service = Ip2proxyRails.new('1.2.3.4') @is_proxy = proxy_service.is_proxy @proxy_type = proxy_service.proxy_type @country_code = proxy_service.country_code @country_name = proxy_service.country_name @region = proxy_service.region @city = proxy_service.city @isp = proxy_service.isp @domain = proxy_service.domain @usage_type = proxy_service.usagetype @asn = proxy_service.asn @as = proxy_service.as @last_seen = proxy_service.last_seen @threat = proxy_service.threat @provider = proxy_service.provider @fraud_score = proxy_service.fraud_score end end
- Open the app/views/test/index.html.erb in any text editor and add the below lines into it.
<p>is Proxy: <%= @is_proxy %></p> <p>Proxy Type: <%= @proxy_type %></p> <p>Country Code: <%= @country_code %></p> <p>Country Name: <%= @country_name %></p> <p>Region Name: <%= @region %></p> <p>City Name: <%= @city %></p> <p>ISP Name: <%= @isp %></p> <p>Domain Name: <%= @domain %></p> <p>Usage Type: <%= @usage_type %></p> <p>ASN: <%= @asn %></p> <p>AS: <%= @as %></p> <p>Last Seen: <%= @last_seen %></p> <p>Threat: <%= @threat %></p> <p>Provider: <%= @provider %></p> <p>Fraud Score: <%= @fraud_score %></p>
- Add the following line into the config/routes.rb file after the Rails.application.routes.draw do line.
get "/test", to: "test#index"
- Restart your development server.
$ bin/rails server
- Enter the URL /test and run. You should see the proxy information of 1.2.3.4 IP address.
- Done.
By the end of this tutorial, you will be able to perform proxy IP lookups using the IP2Proxy library within the Ruby on Rails framework. You’ll learn how to extract detailed proxy information associated with an IP address, which can provide insights into a user’s geographical location and network details. This data can be valuable for various purposes, such as enhancing data analytics, detecting potential proxy usage for security monitoring and improving user experience by understanding regional access patterns.