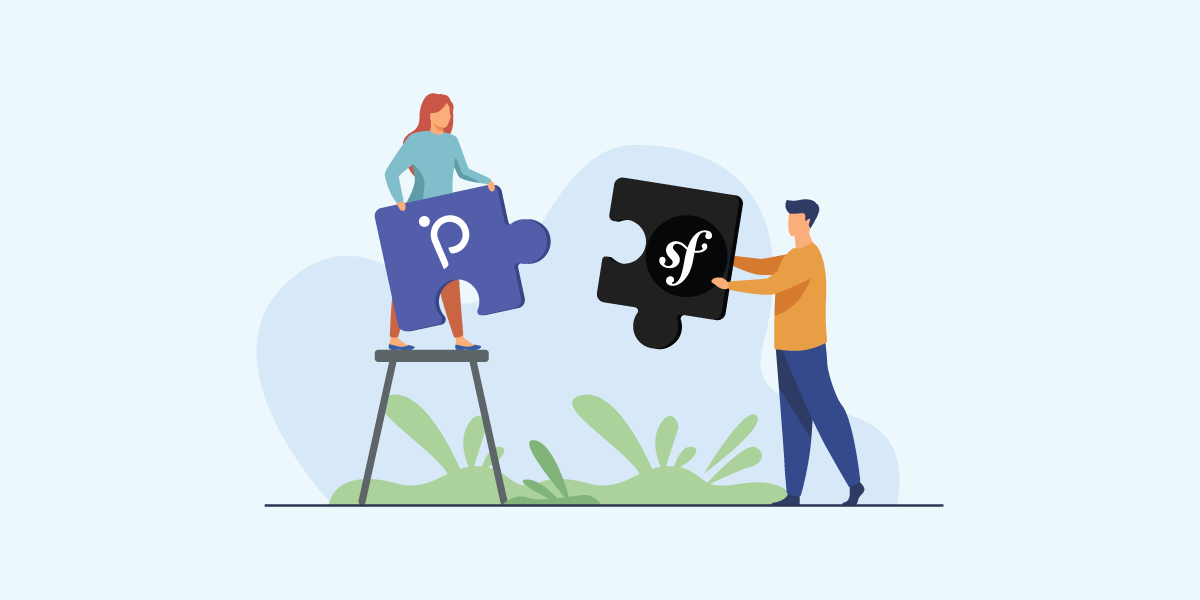
Symfony is a PHP web application framework that is free to use and open-source. It was designed to help in speeding up the web application creation, design and maintenance. It comes with a set of reusable PHP components to help developers in developing web application, for example, form handling and database access. To add more functionality to the web application, developers also install community created and maintained PHP components.
These community created and maintained PHP components are typically open-source. The source codes are easily found on platforms such as GitHub or GitLab. Those components usually have been published to the Packagist repository. Developers can install them through the most popular PHP package manager called Composer.
In this tutorial, we are going to guide you on how to use IP2Location.io PHP SDK in Symfony to retrieve and display geolocation information, and page redirection by the visitor’s IP address. IP2Location.io PHP SDK is a PHP package that allows users to retrieve a set of geolocation information for an IP address. The information includes country, region, district, city, latitude, longitude, ZIP code, time zone, ASN, ISP, domain, net speed, IDD code, area code, weather station data, MNC, MCC, mobile brand, elevation, usage type, address type, advertisement category and proxy data. The component supports both IPv4 and IPv6 lookup.
Before we get started, please make sure that you have properly installed and set up Symfony and Composer. The IP2Location.io PHP SDK requires an API key to function. You may sign up for a free API key at https://www.ip2location.io/pricing. Do note that the continent, country, region and city translations are only available in the Plus and Security plans.
Displaying Geolocation Information
- First of all, if you have not installed the IP2Location.io PHP SDK, you can run the following command in the console to do so:
composer require ip2location/ip2location-io-php
- After that, create a controller file called GeolocationController.php in your symfony_project_root_directory/src/Controller/. Open the file in any text editor.
- Paste the following content into the file:
<?php namespace App\Controller; use Symfony\Component\HttpFoundation\Response; use Symfony\Bundle\FrameworkBundle\Controller\AbstractController; class GeolocationController extends AbstractController { #[Route('/geolocation', name: 'geolocation')] public function display(): Response { // Configures IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new \IP2LocationIO\IPGeolocation($config); try { // Lookup IP address geolocation data $result = $ip2locationio->lookup('8.8.8.8'); // $result->is_proxy = ($result->is_proxy) ? 'TRUE' : 'FALSE'; $result_response = 'IP Address : ' . $result->ip . "<br>"; $result_response .= 'Country Code : ' . $result->country_code . "<br>"; $result_response .= 'Country Name : ' . $result->country_name . "<br>"; $result_response .= 'Region Name : ' . $result->region_name . "<br>"; $result_response .= 'City Name : ' . $result->city_name . "<br>"; $result_response .= 'City Latitude : ' . $result->latitude . "<br>"; $result_response .= 'City Longitude : ' . $result->longitude . "<br>"; $result_response .= 'ZIP Code : ' . $result->zip_code . "<br>"; $result_response .= 'Time Zone : ' . $result->time_zone . "<br>"; $result_response .= 'ASN : ' . $result->asn . "<br>"; $result_response .= 'AS : ' . $result->as . "<br>"; $result_response .= 'Is Proxy : ' . (($result->is_proxy) ? 'TRUE' : 'FALSE') . "<br>"; $result_response .= 'ISP Name : ' . ($result->isp ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Domain Name : ' . ($result->domain ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Net Speed : ' . ($result->net_speend ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'IDD Code : ' . ($result->idd_code ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Area Code : ' . ($result->area_code ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Weather Station Code : ' . ($result->weather_station_code ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Weather Station Name : ' . ($result->weather_station_name ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'MCC : ' . ($result->mcc ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'MNC : ' . ($result->mnc ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Mobile Carrier : ' . ($result->mobile_brand ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Elevation : ' . ($result->elevation ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Usage Type : ' . ($result->usage_type ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Address Type : ' . ($result->address_type ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Category Code : ' . ($result->ads_category ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'Category Name : ' . ($result->ads_category_name ?? 'FIELD NOT SUPPORTED') . "<br>"; $result_response .= 'District Name : ' . ($result->district ?? 'FIELD NOT SUPPORTED') . "<br>"; if (isset($result->continent)) { $result_response .= 'Continent Name : ' . $result->continent->name . "<br>"; $result_response .= 'Continent Code : ' . $result->continent->code . "<br>"; $result_response .= 'Continent Hemisphere : ' . (implode(", ", $result->continent->hemisphere)) . "<br>"; $result_response .= 'Continent Translation : ' . $result->continent->translation->value . ' (' . $result->continent->translation->lang . ')' . "<br>"; } if (isset($result->country)) { $result_response .= 'Country Name : ' . $result->country->name . "<br>"; $result_response .= 'Country Alpha 3 Code : ' . $result->country->alpha3_code . "<br>"; $result_response .= 'Country Numeric Code : ' . $result->country->numeric_code . "<br>"; $result_response .= 'Country Demonym : ' . $result->country->demonym . "<br>"; $result_response .= 'Country Flag : ' . $result->country->flag . "<br>"; $result_response .= 'Country Capital : ' . $result->country->capital . "<br>"; $result_response .= 'Country Total Area : ' . $result->country->total_area . "<br>"; $result_response .= 'Country Population : ' . $result->country->population . "<br>"; $result_response .= 'Country Currency Code : ' . $result->country->currency->code . "<br>"; $result_response .= 'Country Currency Name : ' . $result->country->currency->name . ' (' . $result->country->currency->symbol . ')' . "<br>"; $result_response .= 'Country Language : ' . $result->country->language->name . ' (' . $result->country->language->code . ')' . "<br>"; $result_response .= 'Country TLD : ' . $result->country->tld . "<br>"; $result_response .= 'Country Translation : ' . $result->country->translation->value . ' (' . $result->country->translation->lang . ')' . "<br>"; } if (isset($result->region)) { $result_response .= 'Region Name : ' . $result->region->name . "<br>"; $result_response .= 'Region Code : ' . $result->region->code . "<br>"; $result_response .= 'Region Translation : ' . $result->region->translation->value . ' (' . $result->region->translation->lang . ')' . "<br>"; } if (isset($result->city)) { $result_response .= 'City Name : ' . $result->city->name . "<br>"; $result_response .= 'City Translation : ' . $result->city->translation->value . ' (' . $result->city->translation->lang . ')' . "<br>"; } if (isset($result->time_zone_info)) { $result_response .= 'Olson Time Zone : ' . $result->time_zone_info->olson . "<br>"; $result_response .= 'Current Time : ' . $result->time_zone_info->current_time . "<br>"; $result_response .= 'GMT Offset : ' . $result->time_zone_info->gmt_offset . "<br>"; $result_response .= 'Is DST : ' . (($result->time_zone_info->is_dst)) ? 'TRUE' : 'FALSE' . "<br>"; $result_response .= 'Sunrise Time : ' . $result->time_zone_info->sunrise . "<br>"; $result_response .= 'Sunset Time : ' . $result->time_zone_info->sunset . "<br>"; } if (isset($result->geotargeting)) { $result_response .= 'Metro Code : ' . $result->geotargeting->metro . "<br>"; } if (isset($result->proxy)) { $result_response .= 'Proxy Last Seen : ' . $result->proxy->last_seen . "<br>"; $result_response .= 'Proxy Type : ' . $result->proxy->proxy_type . "<br>"; $result_response .= 'Proxy Threat : ' . $result->proxy->threat . "<br>"; $result_response .= 'Proxy Provider : ' . $result->proxy->provider . "<br>"; } return new Response( '<html><body>' .$result_response. '</body></html>' ); } catch(\Exception $e) { $error_message = $e->getCode() . ": " . $e->getMessage(); return new Response( '<html><body>' .$error_message. '</body></html>' ); } } }
- Next, open the routes.yaml file located at your symfony_project_root_directory/config/ in any text editor, and paste the following contents in a new line:
geolocation: path: /geolocation controller: App\Controller\GeolocationController::display
- Enter the URL /geolocation and run. You should be able to see the information of 8.8.8.8 IP address.
Redirecting user based on its geolocation
- First of all, if you have not installed the IP2Location.io PHP SDK, you can run the following command in the console to do so:
composer require ip2location/ip2location-io-php
- Open your controller file in any text editor.
- Add the following line before the Class:
use Symfony\Component\HttpFoundation\Request;
- Add the following contents in the Class:
public function display(Request $request): Response { /** * @Route("/") */ $ip = $request->getClientIp(); // Configures IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new \IP2LocationIO\IPGeolocation($config); $result = $ip2locationio->lookup($ip); if ($result->country_code == 'US') { return $this->redirect('/'); } }
- Next, open the routes.yaml, and paste the following contents (You can replace the “test” according to your case):
test: path: /test controller: App\Controller\TestController::display
To conclude, you should be able to do an IP geolocation lookup and redirection using the IP2Location.io PHP SDK in the Symfony framework. You can make use of the geolocation information to identify the visitor’s geographical information. Besides, you can also make a proper redirection based on the visitor’s geolocation.
Learn more on how to use IP2Location.io PHP SDK in various frameworks.