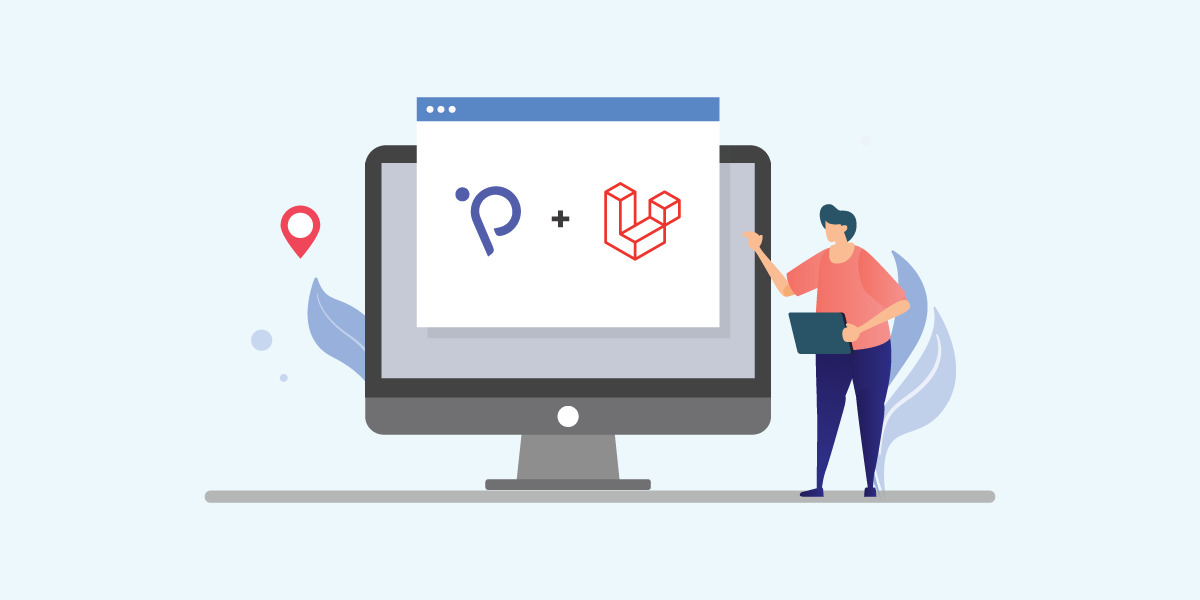
Laravel is a popular PHP web application framework that provides developers with a range of useful tools and features for building modern, scalable, and maintainable applications. One of the key strengths of Laravel is its vibrant ecosystem of third-party packages that extends the framework’s capabilities and makes it easier to build complex applications.
Laravel packages are reusable code modules that developers can install and integrate into their applications to add new functionality or streamline common tasks. These packages are typically published on the Packagist repository and can be installed using Composer, the popular PHP package manager.
IP2Location.io PHP SDK is one of the packages that allows users to query for an enriched data set with an IP address. The information such as country, region, district, city, latitude, longitude, ZIP code, time zone, ASN, ISP, domain, net speed, IDD code, area code, weather station data, MNC, MCC, mobile brand, elevation, usage type, address type, advertisement category and proxy data are included in the enriched data set. It supports both IPv4 and IPv6 addresses lookups.
This tutorial shows you how to use the IP2Location.io PHP SDK in Laravel framework to retrieve the geolocation information for viewing and page redirection.
First of all, we assume that the Laravel framework had been properly set up before following this tutorial. The IP2Location.io PHP SDK requires an API key to function. You may sign up for a free API key at https://www.ip2location.io/pricing. Do note that the continent, country, region and city translations are only available in the Plus and Security plans.
Display Geolocation Data Sample Code
- Run the command below to download the package into the Laravel platform
composer require ip2location/ip2location-io-php
- Create a TestController in Laravel using the command
php artisan make:controller TestController
- Open the app/Http/Controllers/TestController.php in any text editor.
- Add the below lines into the controller file to use IP2Location.io PHP SDK:
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class TestController extends Controller { // Create a lookup function for display public function lookup(){ // Configure IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new \IP2LocationIO\IPGeolocation($config); try { // Lookup IP address geolocation data $result = $ip2locationio->lookup('8.8.8.8'); echo 'IP Address : ' . $result->ip . "<br>"; echo 'Country Code : ' . $result->country_code . "<br>"; echo 'Country Name : ' . $result->country_name . "<br>"; echo 'Region Name : ' . $result->region_name . "<br>"; echo 'City Name : ' . $result->city_name . "<br>"; echo 'City Latitude : ' . $result->latitude . "<br>"; echo 'City Longitude : ' . $result->longitude . "<br>"; echo 'ZIP Code : ' . $result->zip_code . "<br>"; echo 'Time Zone : ' . $result->time_zone . "<br>"; echo 'ASN : ' . $result->asn . "<br>"; echo 'AS : ' . $result->as . "<br>"; echo 'Is Proxy : '; echo ($result->is_proxy) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'ISP Name : ' . ($result->isp ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Domain Name : ' . ($result->domain ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Net Speed : ' . ($result->net_speend ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'IDD Code : ' . ($result->idd_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Area Code : ' . ($result->area_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Code : ' . ($result->weather_station_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Name : ' . ($result->weather_station_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MCC : ' . ($result->mcc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MNC : ' . ($result->mnc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Mobile Carrier : ' . ($result->mobile_brand ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Elevation : ' . ($result->elevation ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Usage Type : ' . ($result->usage_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Address Type : ' . ($result->address_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Code : ' . ($result->ads_category ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Name : ' . ($result->ads_category_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'District Name : ' . ($result->district ?? 'FIELD NOT SUPPORTED') . "<br>"; if (isset($result->continent)) { echo 'Continent Name : ' . $result->continent->name . "<br>"; echo 'Continent Code : ' . $result->continent->code . "<br>"; echo 'Continent Hemisphere : '; print_r($result->continent->hemisphere); echo "<br>"; echo 'Continent Translation : ' . $result->continent->translation->value . ' (' . $result->continent->translation->lang . ')' . "<br>"; } if (isset($result->country)) { echo 'Country Name : ' . $result->country->name . "<br>"; echo 'Country Alpha 3 Code : ' . $result->country->alpha3_code . "<br>"; echo 'Country Numeric Code : ' . $result->country->numeric_code . "<br>"; echo 'Country Demonym : ' . $result->country->demonym . "<br>"; echo 'Country Flag : ' . $result->country->flag . "<br>"; echo 'Country Capital : ' . $result->country->capital . "<br>"; echo 'Country Total Area : ' . $result->country->total_area . "<br>"; echo 'Country Population : ' . $result->country->population . "<br>"; echo 'Country Currency Code : ' . $result->country->currency->code . "<br>"; echo 'Country Currency Name : ' . $result->country->currency->name . ' (' . $result->country->currency->symbol . ')' . "<br>"; echo 'Country Language : ' . $result->country->language->name . ' (' . $result->country->language->code . ')' . "<br>"; echo 'Country TLD : ' . $result->country->tld . "<br>"; echo 'Country Translation : ' . $result->country->translation->value . ' (' . $result->country->translation->lang . ')' . "<br>"; } if (isset($result->region)) { echo 'Region Name : ' . $result->region->name . "<br>"; echo 'Region Code : ' . $result->region->code . "<br>"; echo 'Region Translation : ' . $result->region->translation->value . ' (' . $result->region->translation->lang . ')' . "<br>"; } if (isset($result->city)) { echo 'City Name : ' . $result->city->name . "<br>"; echo 'City Translation : ' . $result->city->translation->value . ' (' . $result->city->translation->lang . ')' . "<br>"; } if (isset($result->time_zone_info)) { echo 'Olson Time Zone : ' . $result->time_zone_info->olson . "<br>"; echo 'Current Time : ' . $result->time_zone_info->current_time . "<br>"; echo 'GMT Offset : ' . $result->time_zone_info->gmt_offset . "<br>"; echo 'Is DST : '; echo ($result->time_zone_info->is_dst) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'Sunrise Time : ' . $result->time_zone_info->sunrise . "<br>"; echo 'Sunset Time : ' . $result->time_zone_info->sunset . "<br>"; } if (isset($result->geotargeting)) { echo 'Metro Code : ' . $result->geotargeting->metro . "<br>"; } if (isset($result->proxy)) { echo 'Proxy Last Seen : ' . $result->proxy->last_seen . "<br>"; echo 'Proxy Type : ' . $result->proxy->proxy_type . "<br>"; echo 'Proxy Threat : ' . $result->proxy->threat . "<br>"; echo 'Proxy Provider : ' . $result->proxy->provider . "<br>"; } } catch(Exception $e) { var_dump($e->getCode() . ": " . $e->getMessage()); } } } ?>
- Add the following line into the routes/web.php file,
Route::get('test', 'App\Http\Controllers\TestController@lookup');
- Enter the URL /test and run. You should be able to see the information of 8.8.8.8 IP address.
- Done.
Page Redirection Sample Code
- Run the command below to download the package into the Laravel platform
composer require ip2location/ip2location-io-php
- Add the below lines into the routes/web.php file:
Route::get('/', function () { // Configure IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new \IP2LocationIO\IPGeolocation($config); // Get visitor IP address for lookup $result = $ip2locationio->lookup($_SERVER['REMOTE_ADDR']); // Redirect the US visitors to the specific page if ($result->country_code == 'US') { return redirect('/home/dashboard-us'); } else { return redirect('/home/dashboard'); } });
- Done.
At the conclusion of this tutorial, you should be able to do an IP geolocation lookup and redirection using the IP2Location.io PHP SDK in the Laravel framework. The geolocation information obtained can help to identify the user’s geographical information and use it for data analytics and page redirection.
Learn more on how to use IP2Location.io PHP SDK in various frameworks.