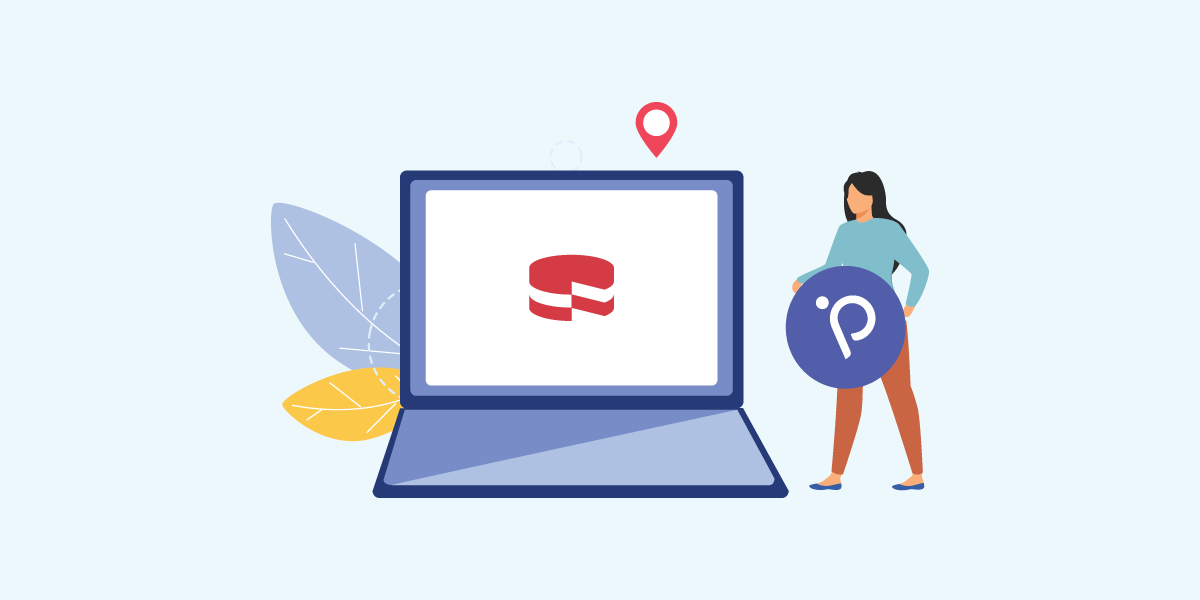
In this article, we will show you how to use IP2Location.io PHP SDK in CakePHP to retrieve the geolocation information and user redirection.
CakePHP is a powerful open-source web framework that is widely used by the developers around the world. It enables the developers to build a powerful yet extendable website by providing them with a set of tools. Other than that, developers also can make use of CakePHP plugins to enhance the website. Those plugins can help to add on more functionalities such as enhanced authentication, integration with external web services and so on.
CakePHP plugins are developed by the community and is available in GitHub or some other public repositories such as Packagist. CakePHP allows developers to use a dependency manager called Composer to install and manage the plugins. This brings much needed convenience to both website developers and plugin developers.
IP2Location.io is a fast and accurate geolocation API to provide the geolocation information to the user. The user can get a variety of information such as the country, region, city, district, latitude, longitude, ZIP code, time zone, ASN, ISP, domain, net speed, IDD code, area code, weather station data, MNC, MCC, mobile brand, elevation, usage type, address type, advertisement category, and proxy data.
Before we get started, you have to ensure that you have an existing CakePHP project to begin with. The IP2Location.io PHP SDK requires an API key to function. You may sign up for a free API key at https://www.ip2location.io/pricing. Do note that the continent, country, region and city translations are only available in the Plus and Security plans.
Displaying Geolocation Information
- First of all, if you have not installed the IP2Location.io PHP SDK, you can run the following command in the console to do so:
composer require ip2location/ip2location-io-php
- Create a template file called view.php in your cakephp_root_dir/templates/Pages/, and open the file in any text editor.
- Paste the following content into the file:
<?php // Configures IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new IP2LocationIO\IPGeolocation($config); ?> <!DOCTYPE html> <html> <head> <?= $this->Html->charset() ?> <meta name="viewport" content="width=device-width, initial-scale=1"> <?= $this->Html->meta('icon') ?> <link href="https://fonts.googleapis.com/css?family=Raleway:400,700" rel="stylesheet"> <?= $this->Html->css(['normalize.min', 'milligram.min', 'cake', 'home']) ?> <?= $this->fetch('meta') ?> <?= $this->fetch('css') ?> <?= $this->fetch('script') ?> </head> <body> Query result for IP address 8.8.8.8:<br/> <?php try { // Lookup IP address geolocation data $result = $ip2locationio->lookup('8.8.8.8'); echo 'IP Address : ' . $result->ip . "<br>"; echo 'Country Code : ' . $result->country_code . "<br>"; echo 'Country Name : ' . $result->country_name . "<br>"; echo 'Region Name : ' . $result->region_name . "<br>"; echo 'City Name : ' . $result->city_name . "<br>"; echo 'City Latitude : ' . $result->latitude . "<br>"; echo 'City Longitude : ' . $result->longitude . "<br>"; echo 'ZIP Code : ' . $result->zip_code . "<br>"; echo 'Time Zone : ' . $result->time_zone . "<br>"; echo 'ASN : ' . $result->asn . "<br>"; echo 'AS : ' . $result->as . "<br>"; echo 'Is Proxy : '; echo ($result->is_proxy) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'ISP Name : ' . ($result->isp ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Domain Name : ' . ($result->domain ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Net Speed : ' . ($result->net_speend ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'IDD Code : ' . ($result->idd_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Area Code : ' . ($result->area_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Code : ' . ($result->weather_station_code ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Weather Station Name : ' . ($result->weather_station_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MCC : ' . ($result->mcc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'MNC : ' . ($result->mnc ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Mobile Carrier : ' . ($result->mobile_brand ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Elevation : ' . ($result->elevation ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Usage Type : ' . ($result->usage_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Address Type : ' . ($result->address_type ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Code : ' . ($result->ads_category ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'Category Name : ' . ($result->ads_category_name ?? 'FIELD NOT SUPPORTED') . "<br>"; echo 'District Name : ' . ($result->district ?? 'FIELD NOT SUPPORTED') . "<br>"; if (isset($result->continent)) { echo 'Continent Name : ' . $result->continent->name . "<br>"; echo 'Continent Code : ' . $result->continent->code . "<br>"; echo 'Continent Hemisphere : '; print_r($result->continent->hemisphere); echo "<br>"; echo 'Continent Translation : ' . $result->continent->translation->value . ' (' . $result->continent->translation->lang . ')' . "<br>"; } if (isset($result->country)) { echo 'Country Name : ' . $result->country->name . "<br>"; echo 'Country Alpha 3 Code : ' . $result->country->alpha3_code . "<br>"; echo 'Country Numeric Code : ' . $result->country->numeric_code . "<br>"; echo 'Country Demonym : ' . $result->country->demonym . "<br>"; echo 'Country Flag : ' . $result->country->flag . "<br>"; echo 'Country Capital : ' . $result->country->capital . "<br>"; echo 'Country Total Area : ' . $result->country->total_area . "<br>"; echo 'Country Population : ' . $result->country->population . "<br>"; echo 'Country Currency Code : ' . $result->country->currency->code . "<br>"; echo 'Country Currency Name : ' . $result->country->currency->name . ' (' . $result->country->currency->symbol . ')' . "<br>"; echo 'Country Language : ' . $result->country->language->name . ' (' . $result->country->language->code . ')' . "<br>"; echo 'Country TLD : ' . $result->country->tld . "<br>"; echo 'Country Translation : ' . $result->country->translation->value . ' (' . $result->country->translation->lang . ')' . "<br>"; } if (isset($result->region)) { echo 'Region Name : ' . $result->region->name . "<br>"; echo 'Region Code : ' . $result->region->code . "<br>"; echo 'Region Translation : ' . $result->region->translation->value . ' (' . $result->region->translation->lang . ')' . "<br>"; } if (isset($result->city)) { echo 'City Name : ' . $result->city->name . "<br>"; echo 'City Translation : ' . $result->city->translation->value . ' (' . $result->city->translation->lang . ')' . "<br>"; } if (isset($result->time_zone_info)) { echo 'Olson Time Zone : ' . $result->time_zone_info->olson . "<br>"; echo 'Current Time : ' . $result->time_zone_info->current_time . "<br>"; echo 'GMT Offset : ' . $result->time_zone_info->gmt_offset . "<br>"; echo 'Is DST : '; echo ($result->time_zone_info->is_dst) ? 'TRUE' : 'FALSE'; echo "<br>"; echo 'Sunrise Time : ' . $result->time_zone_info->sunrise . "<br>"; echo 'Sunset Time : ' . $result->time_zone_info->sunset . "<br>"; } if (isset($result->geotargeting)) { echo 'Metro Code : ' . $result->geotargeting->metro . "<br>"; } if (isset($result->proxy)) { echo 'Proxy Last Seen : ' . $result->proxy->last_seen . "<br>"; echo 'Proxy Type : ' . $result->proxy->proxy_type . "<br>"; echo 'Proxy Threat : ' . $result->proxy->threat . "<br>"; echo 'Proxy Provider : ' . $result->proxy->provider . "<br>"; } } catch(Exception $e) { echo $e->getCode() . ": " . $e->getMessage(); } ?> </body> </html>
- After that, open the routes.php located at your cakephp_root_dir/config/ in any text editor, and paste the following line right after the line
$routes->scope('/', function (RouteBuilder $builder) {
:
$builder->connect('/test1', ['controller' => 'Pages', 'action' => 'display', 'view']);
- This example used the PagesController.php located in cakephp_root_dir/src/Controller/. If you do not have this file, create the same file in the same location and paste the following content into the file:
<?php declare(strict_types=1); /** * CakePHP(tm) : Rapid Development Framework (https://cakephp.org) * Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org) * * Licensed under The MIT License * For full copyright and license information, please see the LICENSE.txt * Redistributions of files must retain the above copyright notice. * * @copyright Copyright (c) Cake Software Foundation, Inc. (https://cakefoundation.org) * @link https://cakephp.org CakePHP(tm) Project * @since 0.2.9 * @license https://opensource.org/licenses/mit-license.php MIT License */ namespace App\Controller; use Cake\Core\Configure; use Cake\Http\Exception\ForbiddenException; use Cake\Http\Exception\NotFoundException; use Cake\Http\Response; use Cake\Http\ServerRequest; use Cake\View\Exception\MissingTemplateException; /** * Static content controller * * This controller will render views from templates/Pages/ * * @link https://book.cakephp.org/4/en/controllers/pages-controller.html */ class PagesController extends AppController { /** * Displays a view * * @param string ...$path Path segments. * @return \Cake\Http\Response|null * @throws \Cake\Http\Exception\ForbiddenException When a directory traversal attempt. * @throws \Cake\View\Exception\MissingTemplateException When the view file could not * be found and in debug mode. * @throws \Cake\Http\Exception\NotFoundException When the view file could not * be found and not in debug mode. * @throws \Cake\View\Exception\MissingTemplateException In debug mode. */ public function display(string ...$path): ?Response { if (!$path) { return $this->redirect('/'); } if (in_array('..', $path, true) || in_array('.', $path, true)) { throw new ForbiddenException(); } $page = $subpage = null; if (!empty($path[0])) { $page = $path[0]; } if (!empty($path[1])) { $subpage = $path[1]; } $this->set(compact('page', 'subpage')); try { return $this->render(implode('/', $path)); } catch (MissingTemplateException $exception) { if (Configure::read('debug')) { throw $exception; } throw new NotFoundException(); } } }
- Enter the URL /view and run. You should be able to see the information of 8.8.8.8 IP address.
Redirecting user based on its geolocation
- First of all, if you have not installed the IP2Location.io PHP SDK, you can run the following command in the console to do so:
composer require ip2location/ip2location-io-php
- Add the below lines into the Controller file that you used for redirection:
// Configure IP2Location.io API key $config = new \IP2LocationIO\Configuration('YOUR_API_KEY'); $ip2locationio = new \IP2LocationIO\IPGeolocation($config); $ip = $this->request->clientIp(); // Get visitor IP address for lookup $result = $ip2locationio->lookup($ip); if ($result->country_code == 'US') { return $this->redirect('/'); }
In conclusion, once you have followed this article, you should be able to query for geolocation information, or redirect user to another page by using IP2Location.io PHP SDK in CakePHP. The geolocation information obtained from the SDK can be used in other cases, for example limiting access to certain page by country or region.
Learn more on how to use IP2Location.io PHP SDK in various frameworks.