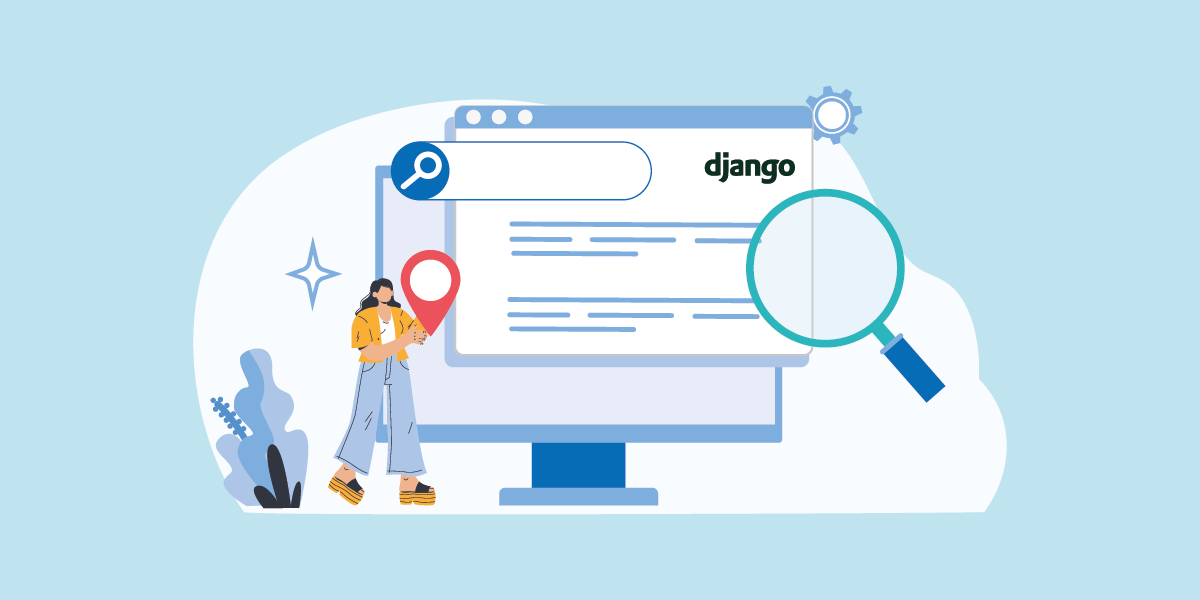
Introduction
Django is a widely-used, free and open source web framework built in Python. It allows web developers to get started to build their website easily. Furthermore, to support their web application development, developers will have numerous external packages to choose from. Those packages can help with improving the user experience and reducing hassle for managing a website. For example, a site admin can use packages to detect where their site visitors are coming from. Such information will help with designing region-specific content. In this article, we will show you how to use IP2Location.io and IP2WHOIS API to perform geolocation and domain WHOIS lookup in Django.
Prerequisite
Before we start, you will be required to install the following components into your server.
- IP2Location.io Python SDK
- To perform geolocation lookup, you need to install it using this command:
pip install ip2location-io
.
- To perform geolocation lookup, you need to install it using this command:
- IP2WHOIS Python SDK
- To perform domain WHOIS lookup, you need to install it using this command:
pip install IP2WHOIS
.
- To perform domain WHOIS lookup, you need to install it using this command:
You will also need a IP2Location.io API key to perform any type of lookup. You can sign up for a free API key, or purchase a plan according to your need.
Steps
- Open your terminal, and create a new Django project by using the following command:
django-admin startproject mysite
- A new folder with the name mysite will be created. Cd to the folder, and type the following command to create a new Django app:
python manage.py startapp myapp
. - We will then start to create the view of the app. Open the mysite/myapp/views.py file, and add the following code into the file:
from django.shortcuts import render from django.template import loader import ip2locationio import ip2whois # Create your views here. from django.http import HttpResponse from django.shortcuts import redirect def get_client_ip(request): x_forwarded_for = request.META.get('HTTP_X_FORWARDED_FOR') if x_forwarded_for: ip = x_forwarded_for.split(',')[0] else: ip = request.META.get('REMOTE_ADDR') return ip def geolocation_lookup(request): template = loader.get_template('myapp/view-ip2locationio-result.html') ip = get_client_ip(request) if ip == '127.0.0.1': # Only define the IP if you are testing on localhost. ip = '8.8.8.8' # Configures IP2Location.io API key configuration = ip2locationio.Configuration('YOUR_API_KEY') ipgeolocation = ip2locationio.IPGeolocation(configuration) rec = ipgeolocation.lookup(ip) context = { 'ip': ip, 'country_code': rec['country_code'], 'country_name': rec['country_name'], 'region_name': rec['region_name'], 'city_name': rec['city_name'], 'latitude': rec['latitude'], 'longitude': rec['longitude'], 'zip_code': rec['zip_code'], 'time_zone': rec['time_zone'], 'asn': rec['asn'], 'asname': rec['as'], 'is_proxy': 'True' if rec['is_proxy'] else 'False', } return HttpResponse(template.render(context, request)) def whois_lookup(request): template = loader.get_template('myapp/view-ip2whois-result.html') domain = "locaproxy.com" # We will use a fixed value for domain name in this tutorial, but you can always change it to accept input from a form or url. # Configures IP2WHOIS API key ip2whois_init = ip2whois.Api('YOUR_API_KEY') # Lookup domain information results = ip2whois_init.lookup(domain) # Convert JSON to Python dictionary # data = json.loads(results) # Pass the data to the template return HttpResponse(template.render(results, request))
- In the same directory, create a new file called urls.py. Open this file, and add the following code into it:
from django.urls import path from . import views urlpatterns = [ path('lookup', views.geolocation_lookup, name='geolocation_lookup'), path('whoislookup', views.whois_lookup, name='whois_lookup'), ]
- We will also need to create a template file for displaying the result. Under the myapp folder, create a new folder called templates, and under the templates folder create another new folder called myapp.
- Inside the mysite/myapp/templates/myapp folder, create two new html files called view-ip2locationio-result.html and view-ip2whois-result.html. Copy the following code into both html files:
<!-- view-ip2locationio-result.html --> <html> <head> <title>View IP2Location.io result</title> </head> <body> <p>IP: {{ip}}</p> <p>Country Code: {{country_code}}</p> <p>Country Name : {{country_name}}</p> <p>Region Name: {{region_name}}</p> <p>City Name: {{city_name}}</p> <p>Latitude: {{latitude}}</p> <p>Longitude: {{longitude}}</p> <p>ZIP Code: {{zip_code}}</p> <p>Time Zone: {{time_zone}}</p> <p>ASN: {{asn}}</p> <p>AS Name: {{asname}}</p> <p>Is Proxy: {{is_proxy}}</p> </body> </html>
<!-- view-ip2whois-result.html --> <!DOCTYPE html> <html> <head> <title>Domain Information: {{ domain }}</title> </head> <body> <h1>Domain Information for {{ domain }}</h1> <p><strong>Domain ID:</strong> {{ domain_id }}</p> <p><strong>Status:</strong> {{ status }}</p> <p><strong>Creation Date:</strong> {{ create_date }}</p> <p><strong>Update Date:</strong> {{ update_date }}</p> <p><strong>Expiration Date:</strong> {{ expire_date }}</p> <p><strong>Domain Age:</strong> {{ domain_age }} days</p> <p><strong>WHOIS Server:</strong> {{ whois_server }}</p> <h2>Registrar Information</h2> <p><strong>IANA ID:</strong> {{ registrar.iana_id }}</p> <p><strong>Name:</strong> {{ registrar.name }}</p> <p><strong>URL:</strong> <a href="{{ registrar.url }}">{{ registrar.url }}</a></p> <h2>Registrant Information</h2> <p><strong>Name:</strong> {{ registrant.name }}</p> <p><strong>Organization:</strong> {{ registrant.organization }}</p> <p><strong>Street Address:</strong> {{ registrant.street_address }}</p> <p><strong>City:</strong> {{ registrant.city }}</p> <p><strong>Region:</strong> {{ registrant.region }}</p> <p><strong>ZIP Code:</strong> {{ registrant.zip_code }}</p> <p><strong>Country:</strong> {{ registrant.country }}</p> <p><strong>Phone:</strong> {{ registrant.phone }}</p> <p><strong>Fax:</strong> {{ registrant.fax }}</p> <p><strong>Email:</strong> <a href="{{ registrant.email }}">{{ registrant.email }}</a></p> <h2>Administrative Contact Information</h2> <p><strong>Name:</strong> {{ admin.name }}</p> <p><strong>Organization:</strong> {{ admin.organization }}</p> <p><strong>Street Address:</strong> {{ admin.street_address }}</p> <p><strong>City:</strong> {{ admin.city }}</p> <p><strong>Region:</strong> {{ admin.region }}</p> <p><strong>ZIP Code:</strong> {{ admin.zip_code }}</p> <p><strong>Country:</strong> {{ admin.country }}</p> <p><strong>Phone:</strong> {{ admin.phone }}</p> <p><strong>Fax:</strong> {{ admin.fax }}</p> <p><strong>Email:</strong> <a href="{{ admin.email }}">{{ admin.email }}</a></p> <h2>Technical Contact Information</h2> <p><strong>Name:</strong> {{ tech.name }}</p> <p><strong>Organization:</strong> {{ tech.organization }}</p> <p><strong>Street Address:</strong> {{ tech.street_address }}</p> <p><strong>City:</strong> {{ tech.city }}</p> <p><strong>Region:</strong> {{ tech.region }}</p> <p><strong>ZIP Code:</strong> {{ tech.zip_code }}</p> <p><strong>Country:</strong> {{ tech.country }}</p> <p><strong>Phone:</strong> {{ tech.phone }}</p> <p><strong>Fax:</strong> {{ tech.fax }}</p> <p><strong>Email:</strong> <a href="{{ tech.email }}">{{ tech.email }}</a></p> <h2>Billing Contact Information</h2> <p><strong>Name:</strong> {{ billing.name }}</p> <p><strong>Organization:</strong> {{ billing.organization }}</p> <p><strong>Street Address:</strong> {{ billing.street_address }}</p> <p><strong>City:</strong> {{ billing.city }}</p> <p><strong>Region:</strong> {{ billing.region }}</p> <p><strong>ZIP Code:</strong> {{ billing.zip_code }}</p> <p><strong>Country:</strong> {{ billing.country }}</p> <p><strong>Phone:</strong> {{ billing.phone }}</p> <p><strong>Fax:</strong> {{ billing.fax }}</p> <p><strong>Email:</strong> {{ billing.email }}</p> <h2>Nameservers</h2> <ul> {% for nameserver in nameservers %} <li>{{ nameserver }}</li> {% endfor %} </ul> </body> </html>
- In order to make sure that the page can be found and loaded, we still need to do some configuration. Open the mysite/mysite/urls.py, and add the following line in the urlpatterns list:
path('myapp/', include('myapp.urls')),
- Open the mysite/mysite/settings.py, and add the following line into the INSTALLED_APPS list:
'myapp',
- Remember to save all the changes. Next, change back to the project root directory and use the following command to start a Django development server:
python manage.py runserver
- Now you can go to your browser, and navigate to http://127.0.0.1:8000/myapp/lookup and http://127.0.0.1:8000/myapp/whoislookup to see the outcome. You will see a similar output displayed in the screenshot below.
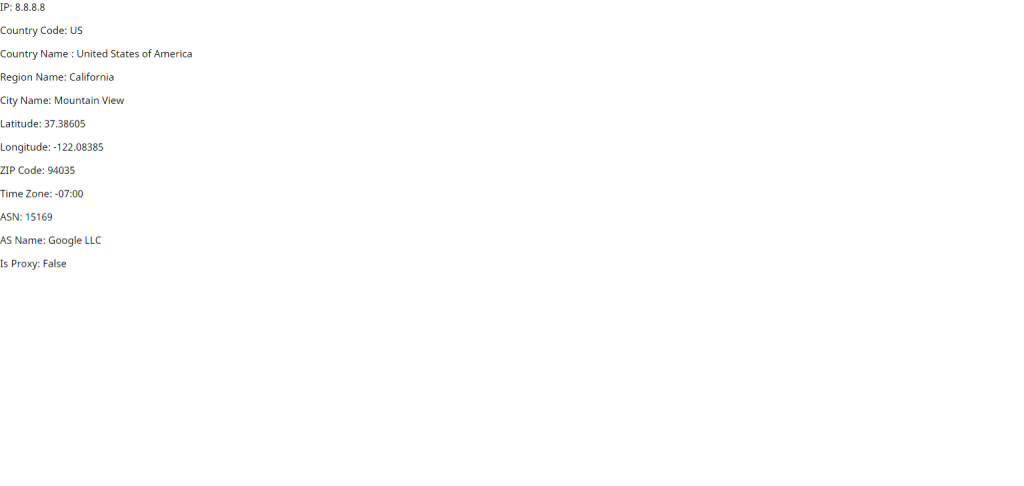
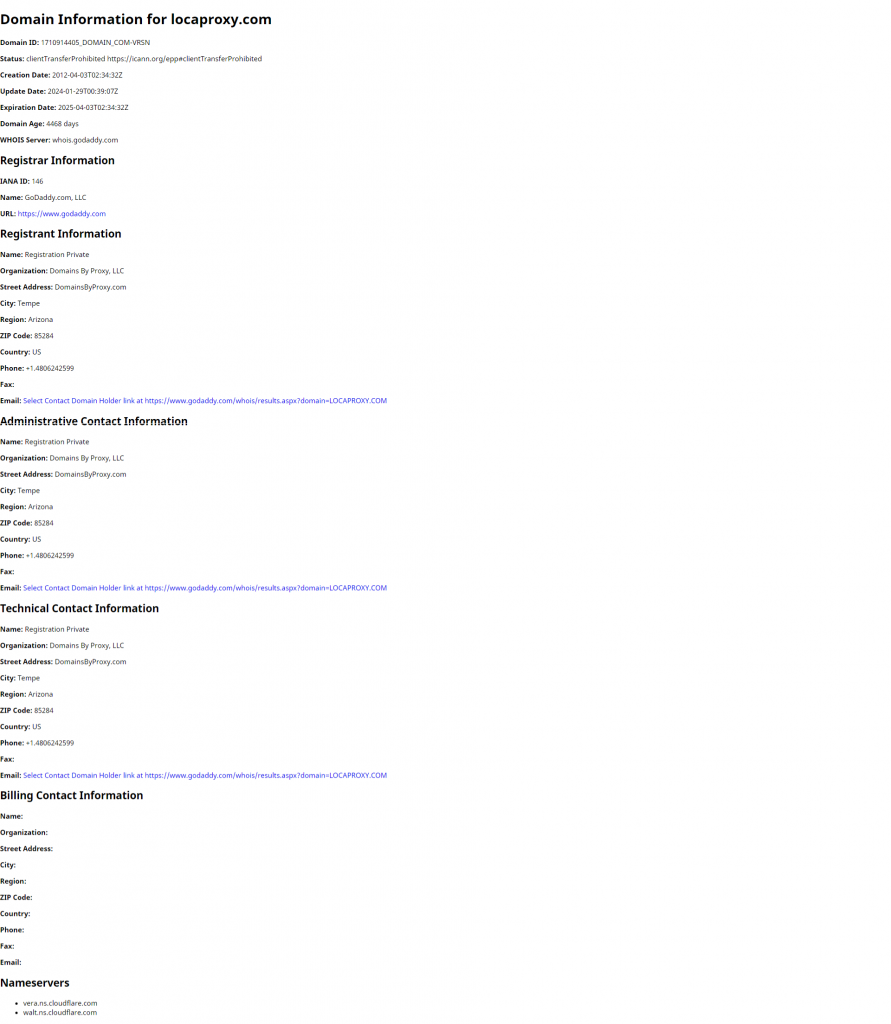