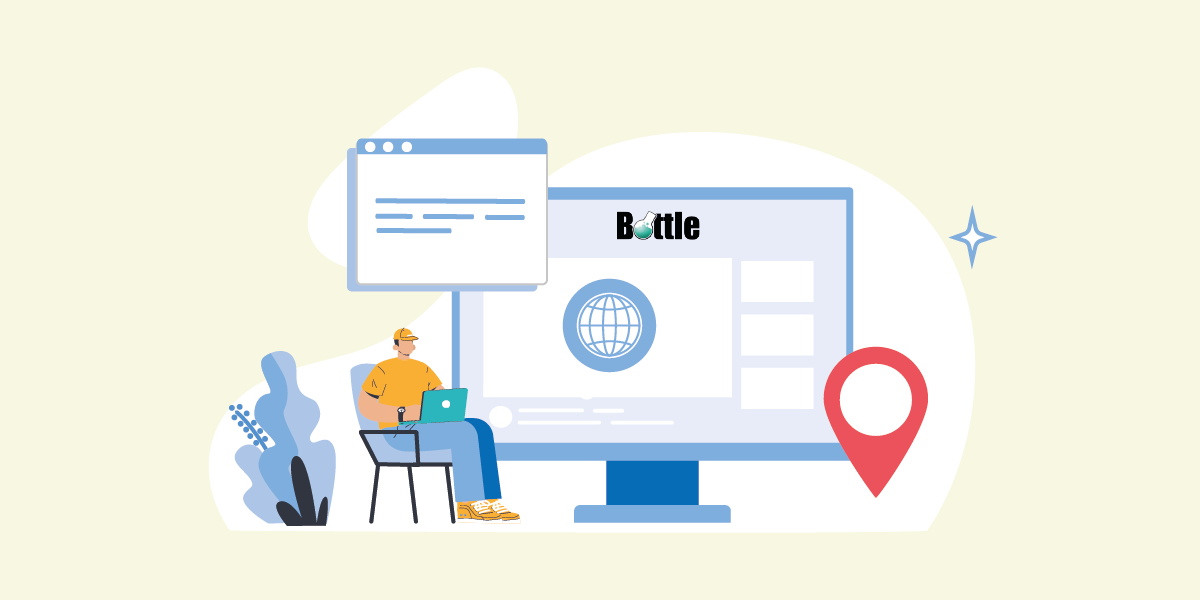
Introduction
Bottle is a micro, simple and fast Python web-framework. It had been distributed as a single file module, and also only rely on Python standard module. Same with other web-frameworks, Bottle allows developers to use third party module to add more functionality to the project. For example, developers can utilize a geolocation lookup extension to lookup for the geolocation information of their visitor. In this article, we will show you how to use IP2Location.io and IP2WHOIS API to perform geolocation and domain WHOIS lookup in Bottle.
Prerequisite
Before we start, you will be required to install the following components into your server.
- IP2Location.io Python SDK
- To perform geolocation lookup, you need to install it using this command:
pip install ip2location-io
.
- To perform geolocation lookup, you need to install it using this command:
- IP2WHOIS Python SDK
- To perform domain WHOIS lookup, you need to install it using this command:
pip install IP2WHOIS
.
- To perform domain WHOIS lookup, you need to install it using this command:
You will also need a IP2Location.io API key to perform any type of lookup. You can sign up for a free API key, or purchase a plan according to your need.
Steps
- In your local server, create a new directory called mywebsite, and navigate to the directory.
- Create a new file called index.py, and paste the following code into the file:
from bottle import Bottle, request, run, template, abort import re import ipaddress import ip2locationio import ip2whois # run(reloader=True) app = Bottle() # Regex pattern for a valid domain name DOMAIN_NAME_PATTERN = re.compile( r'^(?!-)[A-Za-z0-9-]{1,63}(?<!-)\.' r'(?!-)(?:[A-Za-z0-9-]{1,63}\.)*' r'[A-Za-z]{2,6}$' ) @app.route('/geolocation-lookup') @app.route('/geolocation-lookup/<ip>') def geolocation_lookup(ip="127.0.0.1"): try: ipaddress.ip_address(ip) except: abort(400, "Invalid IP address") if ip == '127.0.0.1': if request.environ.get('HTTP_X_FORWARDED_FOR') is None and request.environ.get('REMOTE_ADDR') is not None and request.environ.get('REMOTE_ADDR') != '127.0.0.1': ip = request.environ.get('REMOTE_ADDR') elif request.environ.get('HTTP_X_FORWARDED_FOR') is not None and request.environ.get('HTTP_X_FORWARDED_FOR') != '127.0.0.1': ip = request.environ.get('HTTP_X_FORWARDED_FOR') else: ip = '8.8.8.8' # Google Public IP # Configures IP2Location.io API key configuration = ip2locationio.Configuration('YOUR_API_KEY') ipgeolocation = ip2locationio.IPGeolocation(configuration) rec = ipgeolocation.lookup(ip) return template('display_ip2locationio_result', data=rec) @app.route('/whois-lookup') @app.route('/whois-lookup/<domain>') def whois_lookup(domain="locaproxy.com"): if not DOMAIN_NAME_PATTERN.match(domain): abort(400, "Invalid domain name") # Configures IP2WHOIS API key ip2whois_init = ip2whois.Api('YOUR_API_KEY') # Lookup domain information results = ip2whois_init.lookup(domain) return template('display_ip2whois_result', data=results) def sanitize_domain_name(domain_name): if not DOMAIN_NAME_PATTERN.match(domain_name): abort(400, "Invalid domain name") return domain_name if __name__ == '__main__': run(app, reloader=True, host='localhost', port=8080)
- Create a new sub directory called views. Then, in the views folder, create two new html files called display_ip2locationio_result.html and display_ip2whois_result.html. Paste the following contents into both files respectively:
<!-- display_ip2locationio_result.html --> <!DOCTYPE html> <html> <head> <title>IP Information</title> </head> <body> <h1>IP Information</h1> <ul> <li><strong>IP:</strong> {{data['ip']}}</li> <li><strong>Country Code:</strong> {{data['country_code']}}</li> <li><strong>Country Name:</strong> {{data['country_name']}}</li> <li><strong>Region Name:</strong> {{data['region_name']}}</li> <li><strong>City Name:</strong> {{data['city_name']}}</li> <li><strong>Latitude:</strong> {{data['latitude']}}</li> <li><strong>Longitude:</strong> {{data['longitude']}}</li> <li><strong>Zip Code:</strong> {{data['zip_code']}}</li> <li><strong>Time Zone:</strong> {{data['time_zone']}}</li> <li><strong>ASN:</strong> {{data['asn']}}</li> <li><strong>AS:</strong> {{data['as']}}</li> <li><strong>Is Proxy:</strong> {{data['is_proxy']}}</li> </ul> </body> </html>
<!-- display_ip2whois_result.html --> <!DOCTYPE html> <html> <head> <title>Domain Information</title> </head> <body> <h1>Domain Information</h1> <ul> <li><strong>Domain:</strong> {{data['domain']}}</li> <li><strong>Domain ID:</strong> {{data['domain_id']}}</li> <li><strong>Status:</strong> {{data['status']}}</li> <li><strong>Create Date:</strong> {{data['create_date']}}</li> <li><strong>Update Date:</strong> {{data['update_date']}}</li> <li><strong>Expire Date:</strong> {{data['expire_date']}}</li> <li><strong>Domain Age:</strong> {{data['domain_age']}}</li> <li><strong>Whois Server:</strong> {{data['whois_server']}}</li> </ul> <h2>Registrar</h2> <ul> <li><strong>IANA ID:</strong> {{data['registrar']['iana_id']}}</li> <li><strong>Name:</strong> {{data['registrar']['name']}}</li> <li><strong>URL:</strong> <a href="{{data['registrar']['url']}}">{{data['registrar']['url']}}</a></li> </ul> <h2>Registrant</h2> <ul> <li><strong>Name:</strong> {{data['registrant']['name']}}</li> <li><strong>Organization:</strong> {{data['registrant']['organization']}}</li> <li><strong>Street Address:</strong> {{data['registrant']['street_address']}}</li> <li><strong>City:</strong> {{data['registrant']['city']}}</li> <li><strong>Region:</strong> {{data['registrant']['region']}}</li> <li><strong>Zip Code:</strong> {{data['registrant']['zip_code']}}</li> <li><strong>Country:</strong> {{data['registrant']['country']}}</li> <li><strong>Phone:</strong> {{data['registrant']['phone']}}</li> <li><strong>Fax:</strong> {{data['registrant']['fax']}}</li> <li><strong>Email:</strong> {{data['registrant']['email']}}</li> </ul> <h2>Admin</h2> <ul> <li><strong>Name:</strong> {{data['admin']['name']}}</li> <li><strong>Organization:</strong> {{data['admin']['organization']}}</li> <li><strong>Street Address:</strong> {{data['admin']['street_address']}}</li> <li><strong>City:</strong> {{data['admin']['city']}}</li> <li><strong>Region:</strong> {{data['admin']['region']}}</li> <li><strong>Zip Code:</strong> {{data['admin']['zip_code']}}</li> <li><strong>Country:</strong> {{data['admin']['country']}}</li> <li><strong>Phone:</strong> {{data['admin']['phone']}}</li> <li><strong>Fax:</strong> {{data['admin']['fax']}}</li> <li><strong>Email:</strong> {{data['admin']['email']}}</li> </ul> <h2>Tech</h2> <ul> <li><strong>Name:</strong> {{data['tech']['name']}}</li> <li><strong>Organization:</strong> {{data['tech']['organization']}}</li> <li><strong>Street Address:</strong> {{data['tech']['street_address']}}</li> <li><strong>City:</strong> {{data['tech']['city']}}</li> <li><strong>Region:</strong> {{data['tech']['region']}}</li> <li><strong>Zip Code:</strong> {{data['tech']['zip_code']}}</li> <li><strong>Country:</strong> {{data['tech']['country']}}</li> <li><strong>Phone:</strong> {{data['tech']['phone']}}</li> <li><strong>Fax:</strong> {{data['tech']['fax']}}</li> <li><strong>Email:</strong> {{data['tech']['email']}}</li> </ul> <h2>Billing</h2> <ul> <li><strong>Name:</strong> {{data['billing']['name']}}</li> <li><strong>Organization:</strong> {{data['billing']['organization']}}</li> <li><strong>Street Address:</strong> {{data['billing']['street_address']}}</li> <li><strong>City:</strong> {{data['billing']['city']}}</li> <li><strong>Region:</strong> {{data['billing']['region']}}</li> <li><strong>Zip Code:</strong> {{data['billing']['zip_code']}}</li> <li><strong>Country:</strong> {{data['billing']['country']}}</li> <li><strong>Phone:</strong> {{data['billing']['phone']}}</li> <li><strong>Fax:</strong> {{data['billing']['fax']}}</li> <li><strong>Email:</strong> {{data['billing']['email']}}</li> </ul> <h2>Nameservers</h2> <ul> % for ns in data['nameservers']: <li>{{ns}}</li> % end </ul> </body> </html>
- In your terminal, navigate to the project directory, and run the following command to start the local server: python index.py. After that, you can go to your browser and browse to http://localhost:8080/geolocation-lookup and http://localhost:8080/whois-lookup to see the results. You will see similar outputs displayed in the screenshot below.
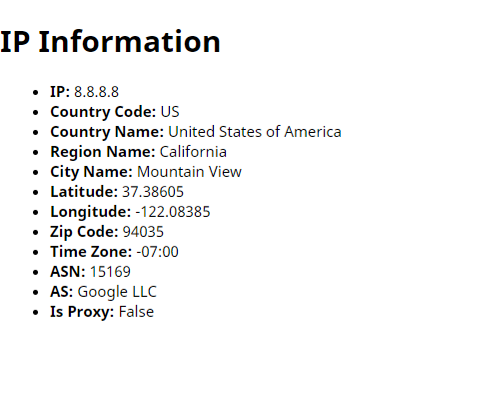
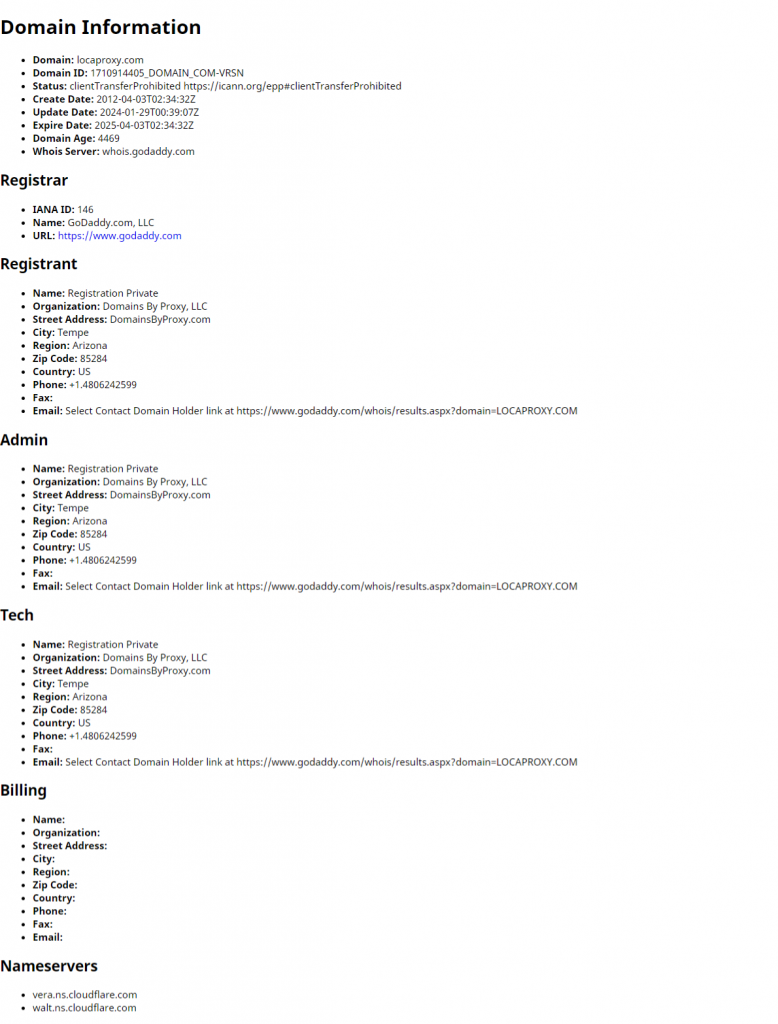