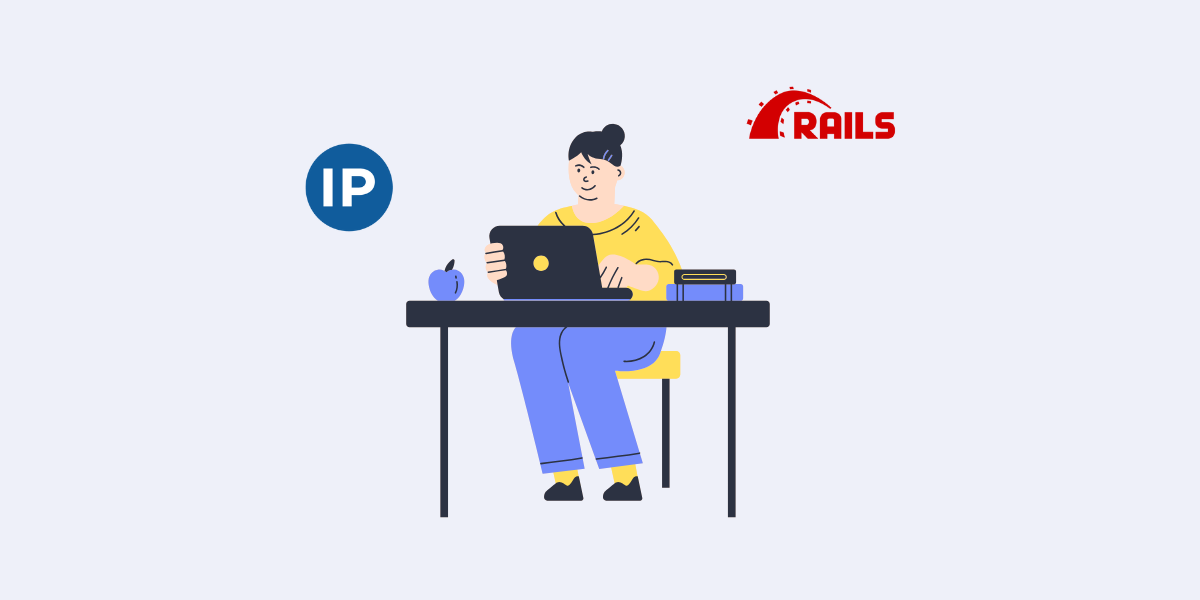
Ruby on Rails, often referred to as Rails, is an open-source web application framework written in the Ruby programming language. It follows the Model-View-Controller (MVC) architectural pattern, which organizes application logic into three interconnected layers: models (data), views (user interface) and controllers (application logic). Rails emphasizes convention over configuration, meaning it provides sensible defaults to minimize the need for extensive setup, speeding up development. Rails is widely used for building scalable, database-driven web applications and is known for its elegant syntax and strong ecosystem.
A Ruby on Rails library, often called a “gem,” is a packaged, reusable code module that extends the functionality of a Rails application. Libraries can provide various functionalities, such as handling authentication, managing payments or interacting with APIs. Gems can be easily integrated into a Rails project via the Gemfile and managed through Bundler, a dependency management tool.
IP2Location Ruby on Rails library is an example of Rails library that enables the user to find the country, region or state, district, city, latitude and longitude, ZIP/Postal code, time zone, Internet Service Provider (ISP) or company name, domain name, net speed, area code, weather station code, weather station name, mobile country code (MCC), mobile network code (MNC) and carrier brand, elevation, usage type, address type, IAB category and ASN from IP address by using IP2Location database. The library reads the geolocation information from IP2Location BIN data file.
This tutorial shows how to use the IP2Location Ruby on Rails library to retrieve the geolocation information. We assume the Ruby on Rails framework had been properly set up before following this tutorial.
Display Geolocation Data Sample Code
- Add this lines to your Ruby on Rails server Gemfile.
gem 'ip2location_ruby'
gem 'ip2location_rails'
- Execute it by running the command.
$ bundle install
- Open the preferred file in the config/environments directory. Add the following code to the chosen configuration file after the Rails.application.configure do line.
config.ip2location_db_path = Rails.root.join('lib', 'assets', 'ip2location_rails', 'IP2LOCATION.BIN').to_s
- Download IP2Location BIN database from https://www.ip2location.com
- Create a folder named as ip2location_rails in the lib/assets directory.
- Unzip and copy the BIN file into lib/assets/ip2location_rails folder.
- Rename the BIN file to IP2LOCATION.BIN.
- Create a TestController using the below command line
bin/rails generate controller Test index --skip-routes
- Open the app/controllers/test_controller.rb in any text editor.
- Add the below lines into the controller file.
require 'ip2location_rails' class TestController < ApplicationController def index location_service = Ip2locationRails.new('8.8.8.8') @country_code = location_service.country_code @country_name = location_service.country_name @region = location_service.region @city = location_service.city @latitude = location_service.latitude @longitude = location_service.longitude @isp = location_service.isp @domain = location_service.domain @netspeed = location_service.netspeed @area_code = location_service.areacode @idd_code = location_service.iddcode @time_zone = location_service.timezone @zip_code = location_service.zipcode @weather_station_name = location_service.weatherstationname @weather_station_code = location_service.weatherstationcode @mcc= location_service.mcc @mnc = location_service.mnc @mobile_brand = location_service.mobilebrand @elevation = location_service.elevation @usage_type = location_service.usagetype @address_type = location_service.addresstype @category = location_service.category @district = location_service.district @asn = location_service.asn @as = location_service.as end end
- Open the app/views/test/index.html.erb in any text editor and add the below lines into it.
<p>Country Code: <%= @country_code %></p> <p>Country Name: <%= @country_name %></p> <p>Region Name: <%= @region %></p> <p>City Name: <%= @city %></p> <p>Latitude: <%= @latitude %></p> <p>Longitude: <%= @longitude %></p> <p>ISP Name: <%= @isp %></p> <p>Domain Name: <%= @domain %></p> <p>Net Speed: <%= @netspeed %></p> <p>Area Code: <%= @area_code %></p> <p>IDD Code: <%= @idd_code %></p> <p>Time Zone: <%= @time_zone %></p> <p>ZIP Code: <%= @zip_code %></p> <p>Weather Station Code: <%= @weather_station_name %></p> <p>Weather Station Name: <%= @weather_station_code %></p> <p>MCC: <%= @mcc %></p> <p>MNC: <%= @mnc %></p> <p>Mobile Carrier: <%= @mobile_brand %></p> <p>Elevation: <%= @elevation %></p> <p>Usage Type: <%= @usage_type %></p> <p>Address Type: <%= @address_type %></p> <p>Category: <%= @category %></p> <p>District: <%= @district %></p> <p>ASN: <%= @asn %></p> <p>AS: <%= @as %></p>
- Add the following line into the config/routes.rb file after the Rails.application.routes.draw do line.
get "/test", to: "test#index"
- Restart your development server.
$ bin/rails server
- Enter the URL /test and run. You should see the information of 8.8.8.8 IP address.
- Done.
By the end of this tutorial, you will have the skills to perform IP geolocation lookups using the IP2Location library within the Ruby on Rails framework. You’ll learn how to retrieve detailed geographical data associated with an IP address, such as the user’s location. This geolocation information can be invaluable for various applications, including data analytics, personalized user experiences and enhancing security measures by understanding user demographics and regional behavior patterns based on their IP addresses.
THE POWER OF IP GEOLOCATION
Find a solution that fits.